How to ingest HL7v2 Messages into Google Cloud
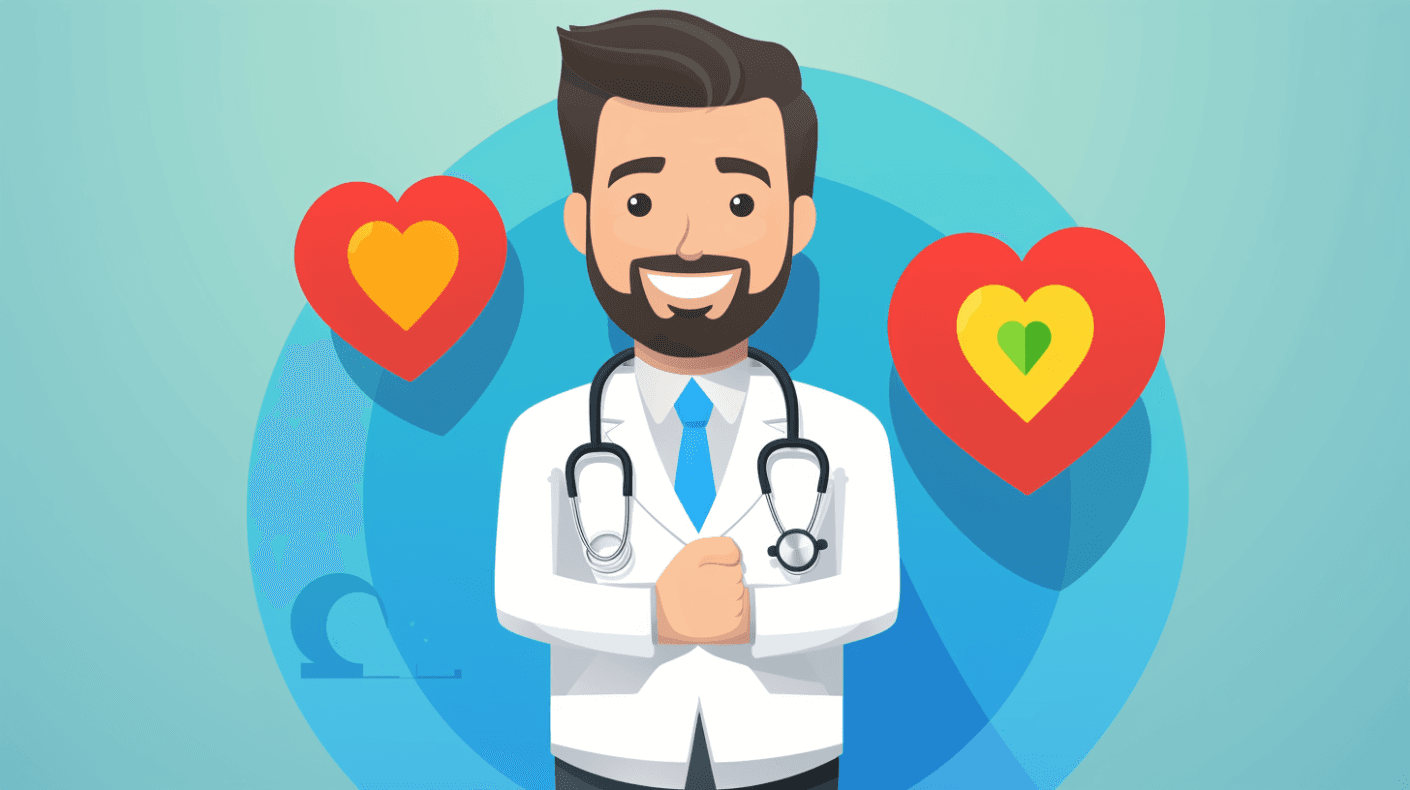
What is HL7v2 again?
HL7v2, which stands for Health Level 7 Version 2, is a messaging standard that has been widely adopted in the healthcare industry since its inception in the late 1980s. It was developed by the international non-profit organization Health Level Seven International (HL7) and has since become the de facto standard for exchanging clinical and administrative data between healthcare systems.
HL7v2 facilitates the seamless exchange of data between different healthcare systems, such as Electronic Health Records (EHRs), Laboratory Information Systems (LIS), and Radiology Information Systems (RIS). This interoperability ensures that patient data is consistent and accessible across different platforms.
Given its longevity and robustness, HL7v2 is used by a vast majority of healthcare institutions worldwide, making it a critical standard to understand and implement.
HL7v2 Message Components
-
Segments: These are the basic units of HL7v2 messages. Each segment starts with a three-character string that identifies the segment type. For instance:
PID
segment contains patient identification information.OBR
segment provides details about the order/request.OBX
segment contains the results or observation.
-
Fields: Within each segment, the data is further divided into fields. Fields are separated by pipe (
|
) characters. For example, in thePID
segment, different fields might contain the patient's first name, last name, date of birth, etc. -
Sub-components: Fields can be further divided into sub-components, which provide even more granular data. Sub-components within a field are typically separated by caret (
^
) characters. For instance, an address field might be broken down into sub-components like street, city, state, and zip code. -
Repeating Fields and Segments: Some fields and segments can repeat within a message. For example, a patient might have multiple phone numbers or addresses.
-
Message Types: HL7v2 messages are categorized into types based on their purpose. Some common message types include:
ADT
(Admit, Discharge, Transfer) - Pertains to patient admission, discharge, transfer, etc.ORM
(Order Message) - Relates to orders for services or goods.ORU
(Observation Result) - Contains results from lab tests or other observations.
-
Trigger Events: Each message type can have multiple trigger events that define the specific action or event that caused the message to be sent. For instance, an
ADT^A01
message indicates a patient admission.
Storing and Managing HL7v2 Messages
HL7v2 messages are the backbone of many healthcare systems, enabling the exchange of crucial clinical and administrative data. Properly storing and managing these messages is vital to ensure data integrity, patient safety, and efficient healthcare operations.
Why Proper Storage Matters
- Data Integrity: Ensuring that messages are stored without alteration is crucial. Any changes, even minor ones, can lead to significant clinical and administrative errors.
- Retrieval Efficiency: Healthcare operations often require rapid access to patient data. Efficient storage solutions ensure that data can be retrieved quickly when needed.
- Compliance: Many jurisdictions have strict regulations about how patient data should be stored, often requiring encryption, backup, and specific retention periods.
Creating a Dataset
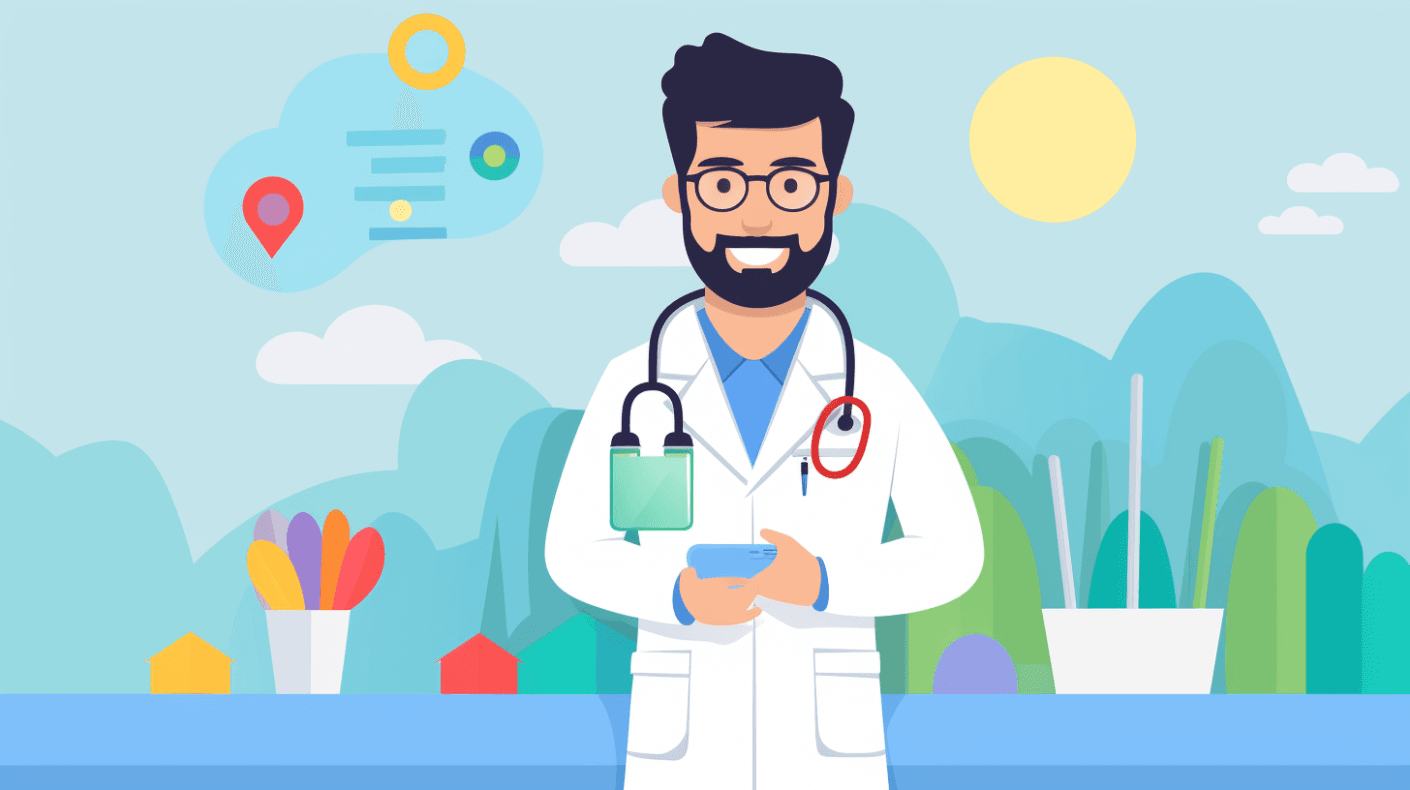
Before storing HL7v2 messages, you need to create a dataset. A dataset in the Google Cloud Healthcare API is a top-level container used to store and analyze data.
from google.cloud import healthcare_v1beta1
client = healthcare_v1beta1.DatasetsClient()
dataset = healthcare_v1beta1.Dataset(
name="projects/{project_id}/locations/{location_id}/datasets/{dataset_id}"
)
response = client.create_dataset(parent="projects/{project_id}/locations/{location_id}", dataset=dataset)
print("Dataset created:", response.name)
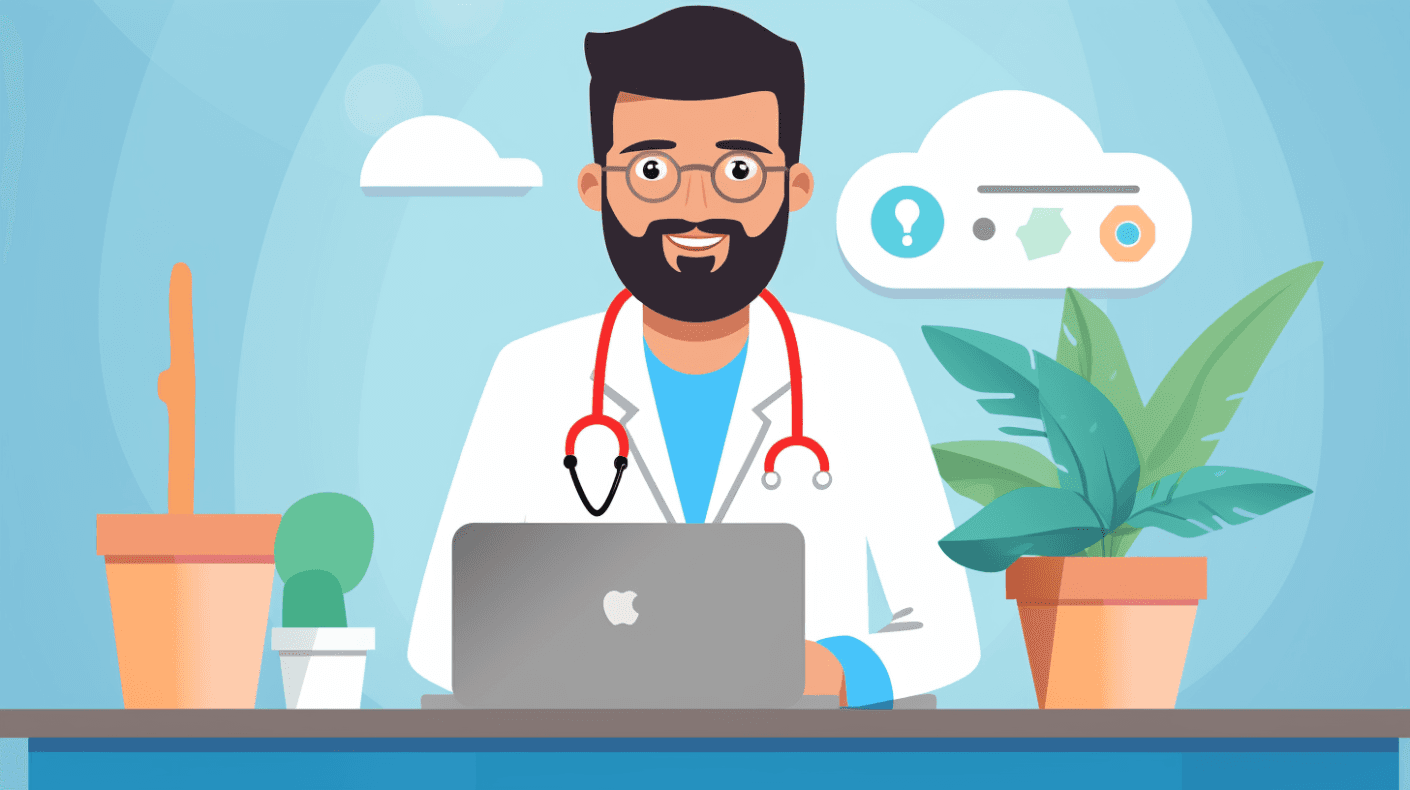
Creating an HL7v2 Store
Once you have a dataset, you can create an HL7v2 store within it. This store is where your HL7v2 messages will be saved.
hl7v2_store = healthcare_v1beta1.Hl7V2Store(name=dataset.name + "/hl7V2Stores/{hl7v2_store_id}")
response = client.create_hl7v2_store(parent=dataset.name, hl7v2_store=hl7v2_store)
print("HL7v2 store created:", response.name)
Storing Messages
With your dataset and HL7v2 store set up, you can now store HL7v2 messages.
message_content = "YOUR_HL7V2_MESSAGE_CONTENT"
message = healthcare_v1beta1.CreateMessageRequest(parent=hl7v2_store.name, message=message_content)
response = client.create_message(request=message)
print("Message stored with ID:", response.name.split('/')[-1])
Retrieving Messages
To retrieve a specific message, you can use its unique ID.
message_name = f"{hl7v2_store.name}/messages/{message_id}"
response = client.get_message(name=message_name)
print("Retrieved message content:", response.data)
Searching for Messages:
You can search for messages based on specific criteria, such as patient ID or message type.
filter_criteria = "YOUR_FILTER_CRITERIA"
response = client.list_messages(parent=hl7v2_store.name, filter=filter_criteria)
for message in response:
print("Message ID:", message.name.split('/')[-1])
Deleting Messages
In some cases, you might need to delete specific messages, either for compliance reasons or to correct errors.
client.delete_message(name=message_name)
print(f"Message {message_name.split('/')[-1]} deleted.")
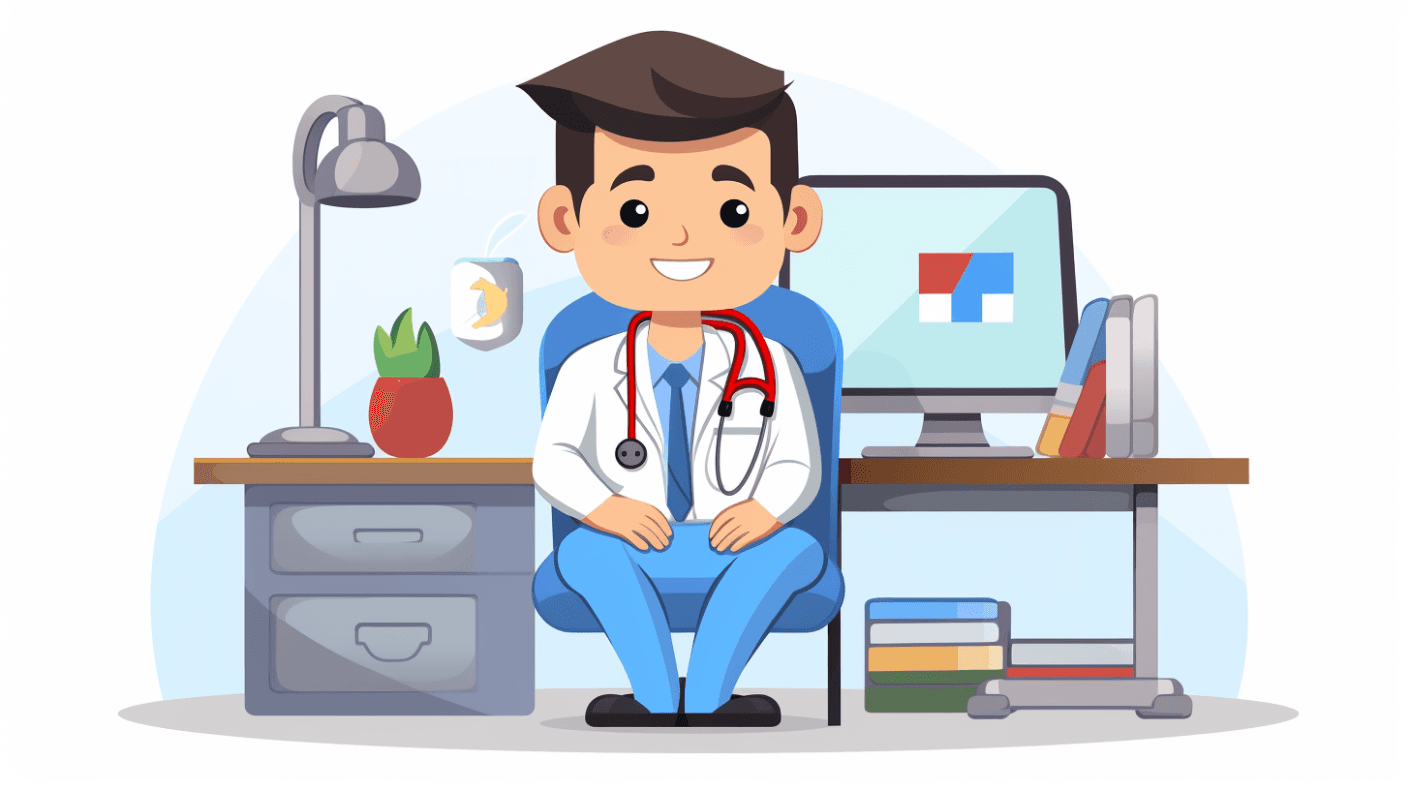
Versioning and Archiving
Given the importance of data integrity in healthcare, it's often necessary to maintain versions of HL7v2 messages. This ensures that any changes or updates to a message can be tracked over time. Google Cloud Healthcare API provides built-in versioning capabilities, allowing you to retrieve previous versions of a message if needed.
Additionally, archiving older messages that are no longer actively used but need to be retained for compliance reasons is crucial. The Google Cloud Healthcare API offers solutions for archiving data, ensuring it's stored securely but can be accessed if needed.
Parsing and Converting HL7v2 Messages
Parsing and converting HL7v2 messages is a fundamental step in processing and understanding the data they contain. Given the structured yet complex nature of HL7v2 messages, specialized tools and methods are required to extract meaningful information from them.
Why Parsing is Essential
- Data Extraction: HL7v2 messages contain a wealth of information, but it's often embedded in a format that's not immediately usable. Parsing allows us to extract specific data elements from these messages.
- Data Transformation: Healthcare systems often need to transform HL7v2 messages into other formats, such as JSON or XML, for further processing or integration with other systems.
- Error Detection: Parsing can help detect errors or inconsistencies in HL7v2 messages, ensuring data quality and integrity.
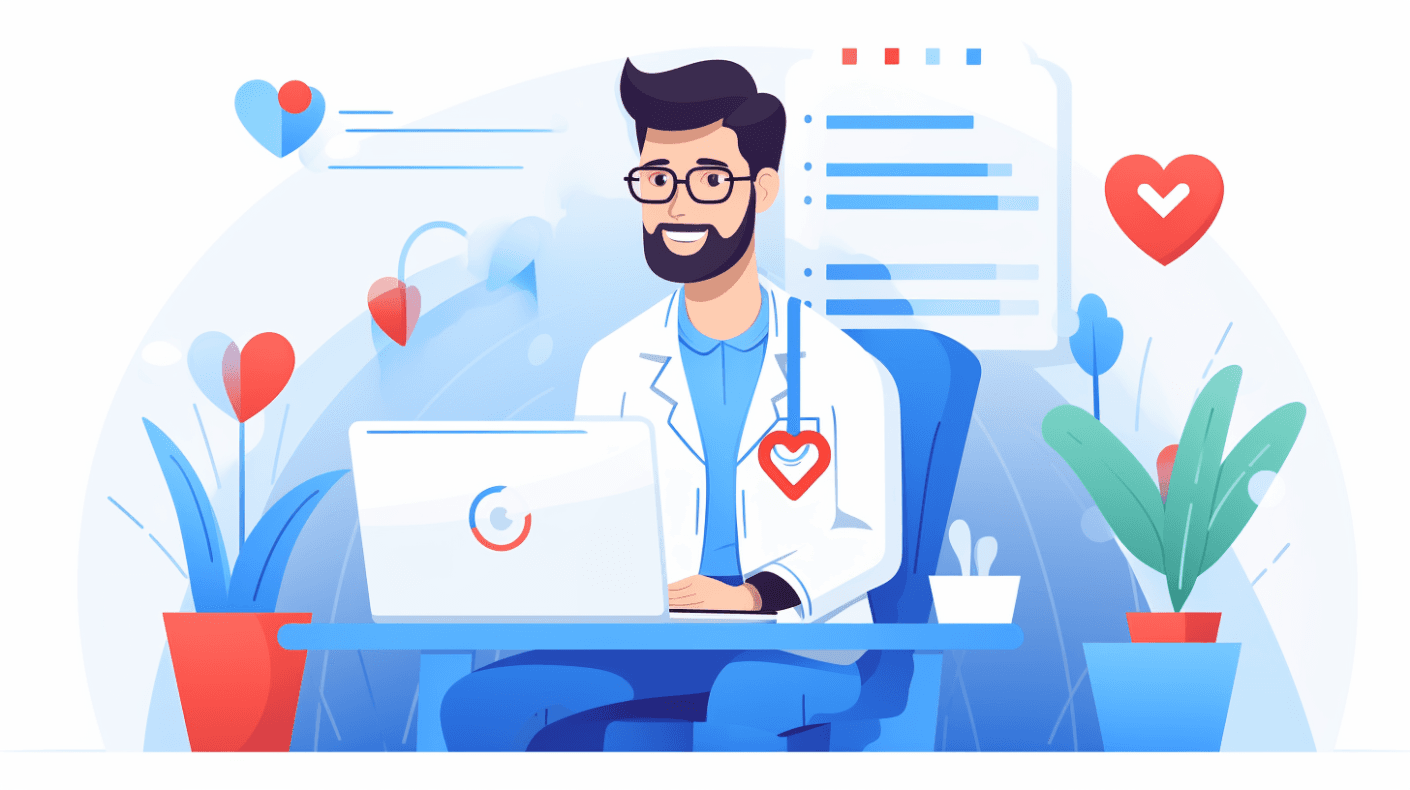
Understanding the Parsing Process
Parsing an HL7v2 message involves breaking it down into its constituent segments, fields, and sub-components. This hierarchical structure is what gives HL7v2 messages their flexibility and power.
Using the Google Cloud Healthcare API for Parsing:
The Google Cloud Healthcare API provides built-in capabilities for parsing HL7v2 messages, making it easier to work with their content.
Parsing a HL7v2 Message
parse_request = healthcare_v1beta1.ParseMessageRequest(name=hl7v2_store.name, message=message_content)
parsed_message = client.parse_message(request=parse_request)
print("Parsed message segments:", parsed_message.segments)
Converting HL7v2 Messages to FHIR
FHIR (Fast Healthcare Interoperability Resources) is a newer, more flexible standard for health data exchange. Converting HL7v2 messages to FHIR resources can make them more accessible and usable in modern healthcare systems.
Conversion Process
- Mapping: Define how data elements in the HL7v2 message map to elements in the FHIR resource. This might involve direct mappings (e.g., patient name to patient name) or more complex transformations.
- Transformation: Use the mappings to transform the HL7v2 message into a FHIR resource.
- Validation: Ensure that the resulting FHIR resource is valid and contains all the required data elements.
Using the Google Cloud Healthcare API for Conversion
conversion_request = healthcare_v1beta1.ConvertMessageRequest(name=hl7v2_store.name, message=message_content)
fhir_resource = client.convert_message(request=conversion_request)
print("Converted FHIR resource:", fhir_resource)
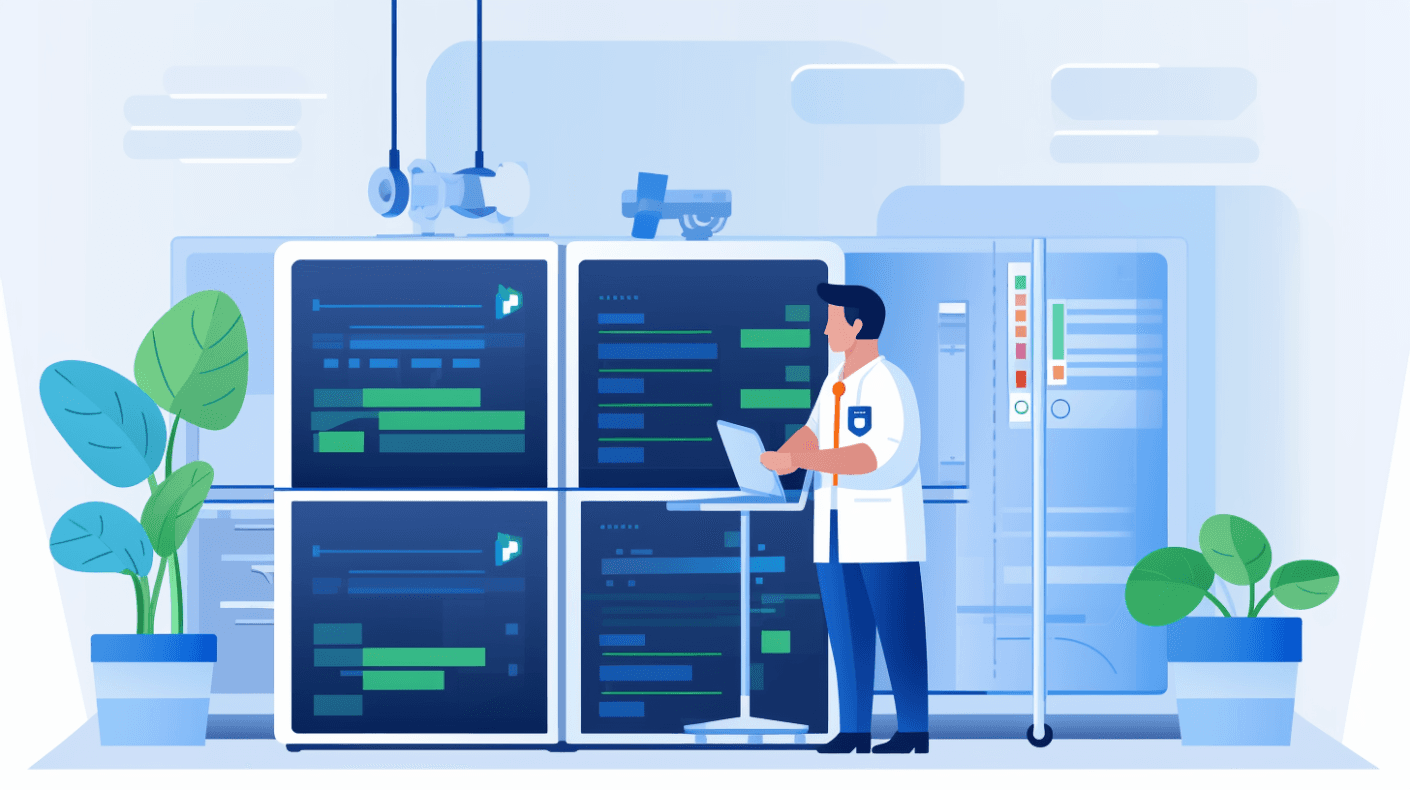
Customizing the Parsing and Conversion Process
While the Google Cloud Healthcare API provides robust default capabilities for parsing and converting HL7v2 messages, there might be cases where you need more customized behavior.
- Custom Schemas: If your HL7v2 messages have a non-standard structure, you can define custom schemas that describe their format. These schemas can then be used by the parser to correctly interpret your messages.
- Custom Mappings: For conversion to FHIR, you can define custom mappings that specify how data elements in your HL7v2 messages should be transformed into FHIR resources.
- Error Handling: Define custom error handling logic to deal with issues that might arise during the parsing or conversion process, such as missing data elements or invalid values.
Using the Default Parser for HL7v2 Messages
The Google Cloud Healthcare API offers a default parser for HL7v2 messages. This parser is designed to handle standard HL7v2 messages, breaking them down into their constituent parts for easier processing and analysis.
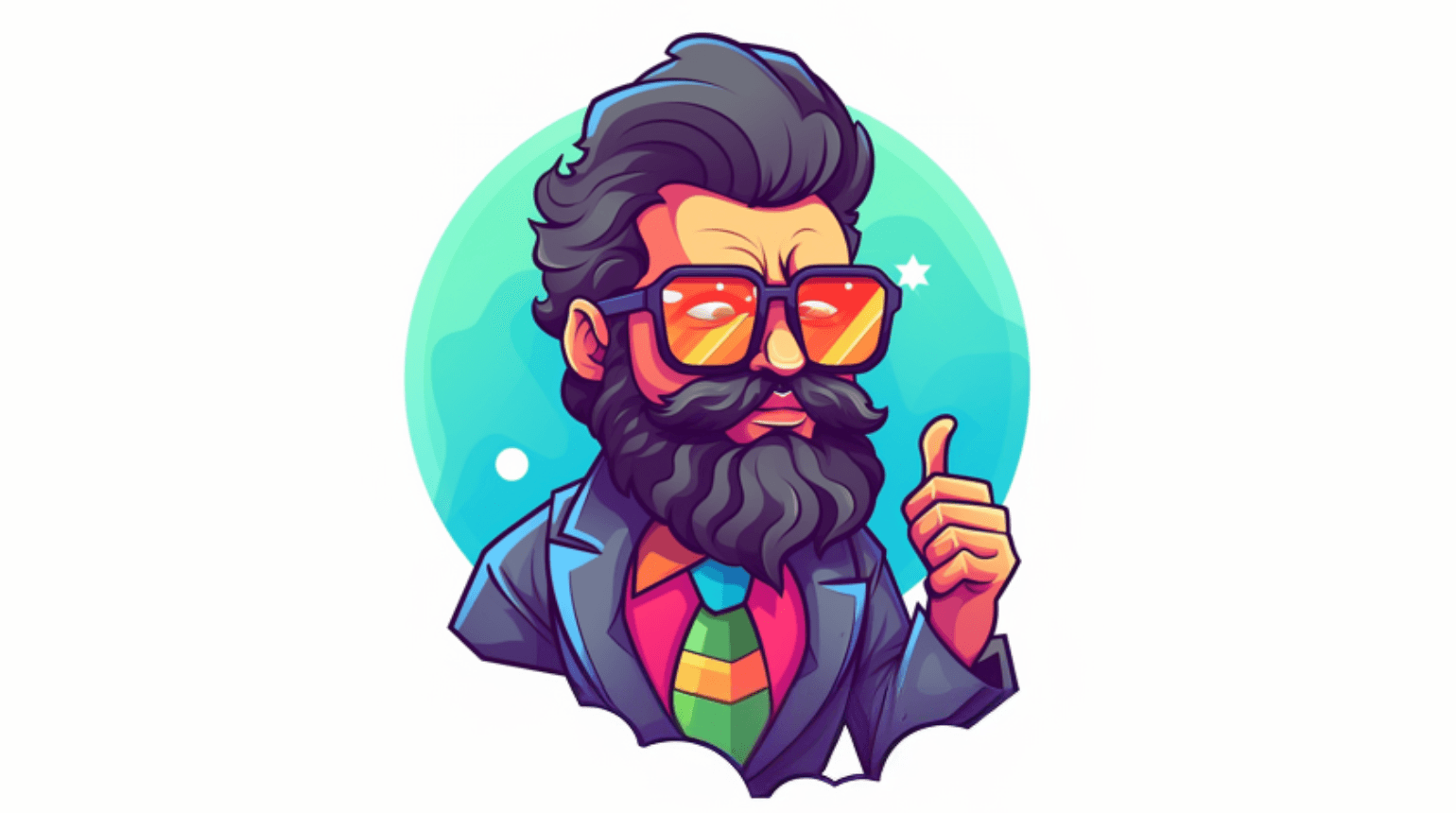
Advantages of the Default Parser
- Ease of Use: The default parser is ready to use out-of-the-box, requiring no additional configuration or setup. This makes it an excellent choice for healthcare systems that use standard HL7v2 messages.
- Accuracy: Built on years of expertise and industry knowledge, the default parser is highly accurate, ensuring that HL7v2 messages are parsed correctly every time.
- Performance: Optimized for speed, the default parser can handle large volumes of HL7v2 messages, making it suitable for busy healthcare systems.
How the Default Parser Works
- Segmentation: The parser first breaks the HL7v2 message into its individual segments, using the carriage return (
\r
) as the delimiter. - Field Extraction: Within each segment, the parser identifies individual fields, using the pipe (
|
) character as the delimiter. - Sub-component Identification: For fields that contain sub-components, the parser further breaks them down, typically using the caret (
^
) character as the delimiter. - Data Type Recognition: The parser identifies the data type of each field, such as string, date, or coded element. This ensures that the data is correctly interpreted and processed.
Using the Default Parser with the Google Cloud Healthcare API
To use the default parser, you simply send your HL7v2 message to the Healthcare API's parsing endpoint. The API will then return the parsed message in a structured format, such as JSON.
parse_request = healthcare_v1beta1.ParseMessageRequest(name=hl7v2_store.name, message=message_content)
parsed_message = client.parse_message(request=parse_request)
print("Parsed message:", parsed_message)
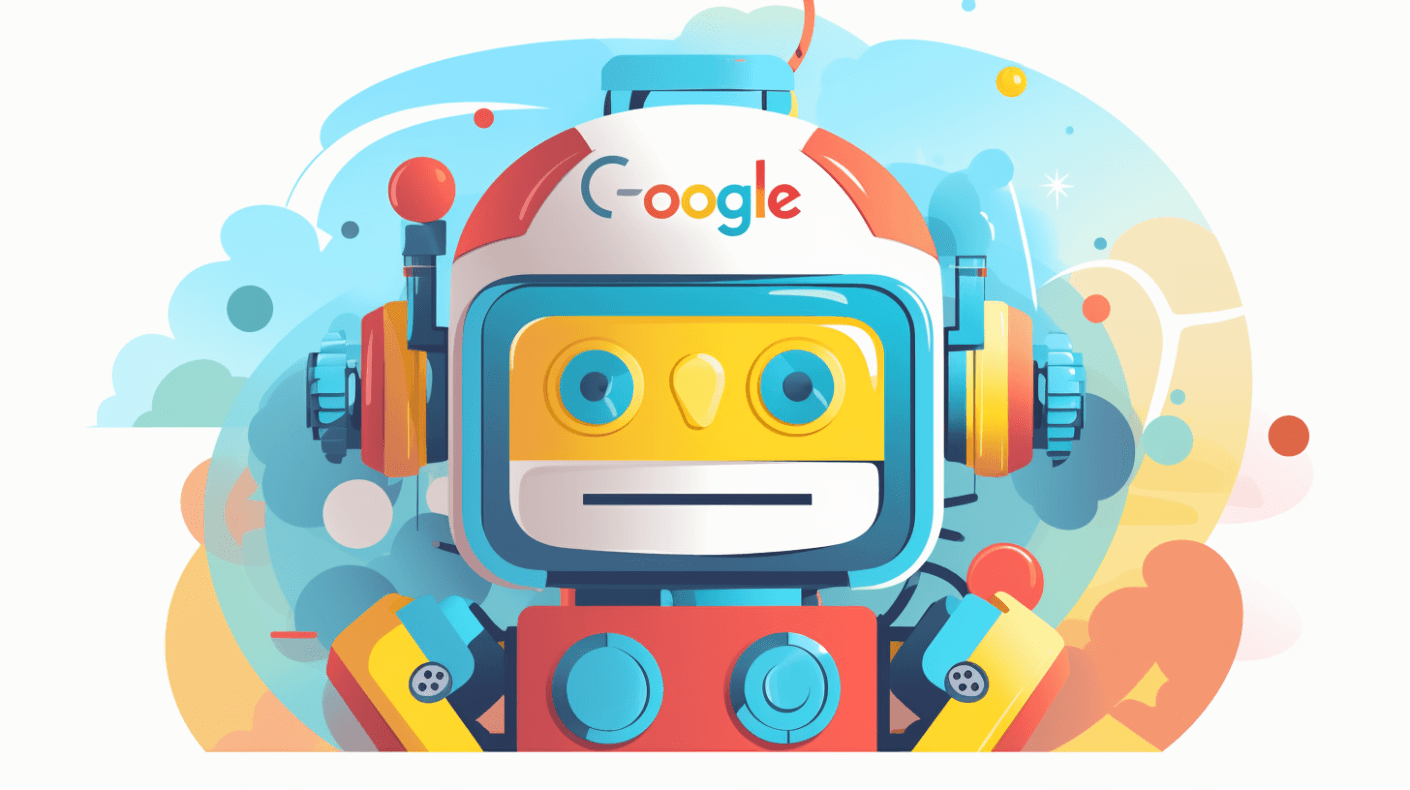
Handling Parsing Errors
While the default parser is highly accurate, there may be instances where it encounters errors or ambiguities in the HL7v2 message. In such cases:
- Error Reporting: The parser will return an error message, detailing the nature of the error and its location in the HL7v2 message.
- Manual Review: It's advisable to review any messages that result in parsing errors manually. This ensures that no critical data is lost or misinterpreted.
- Customization: If you frequently encounter parsing errors due to non-standard HL7v2 message formats, consider customizing the parser or providing additional schemas to better handle your specific messages.
When to Consider Other Parsing Options
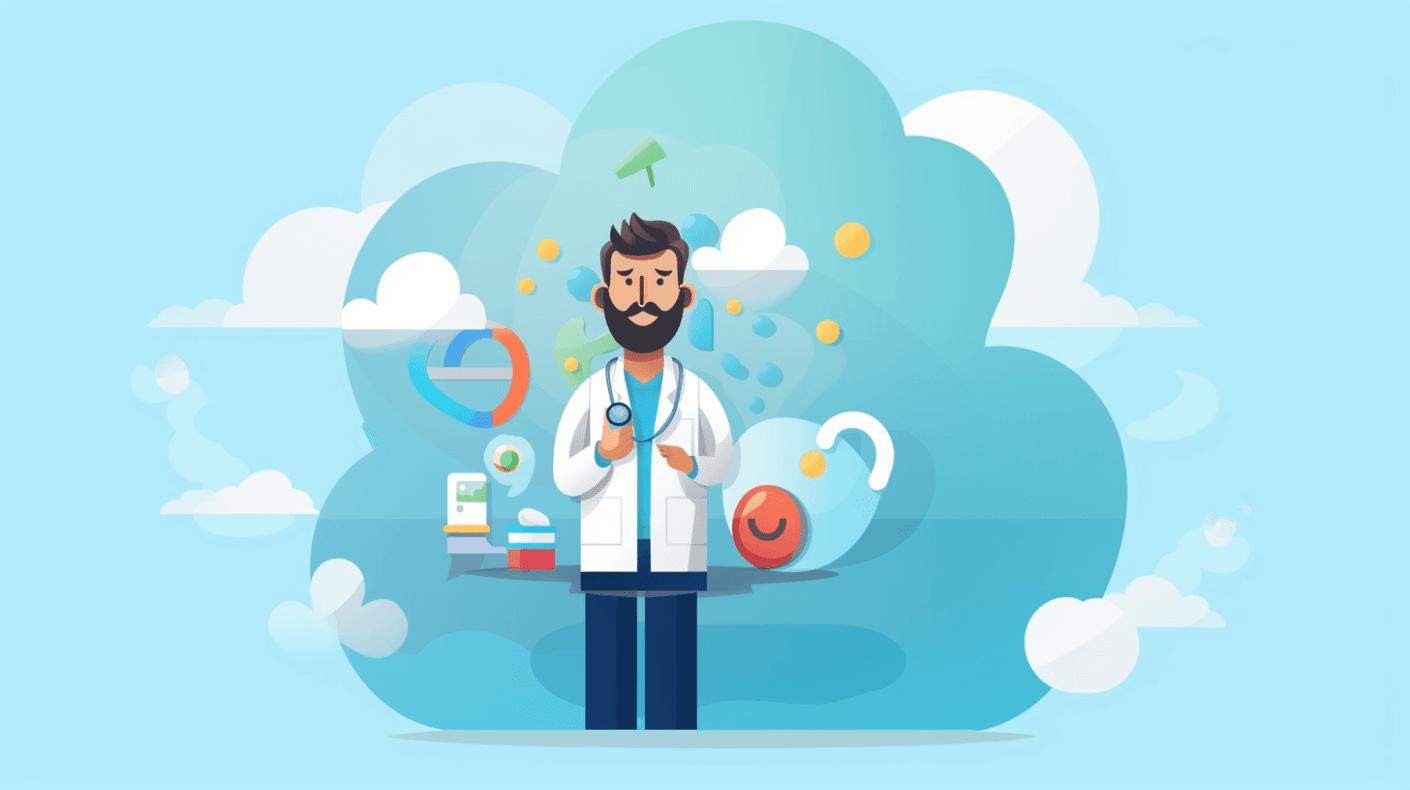
While the default parser is powerful and suitable for many scenarios, there might be situations where you need more advanced or customized parsing capabilities. For instance:
- Non-standard HL7v2 Formats: If your healthcare system uses a non-standard HL7v2 message format, the default parser might not interpret it correctly.
- Custom Data Extraction Needs: If you need to extract specific data elements from HL7v2 messages in a particular way, a customized parser might be more appropriate.
Using a Custom Parser for HL7v2 Messages
While the default parser provided by the Google Cloud Healthcare API is robust and suitable for many standard HL7v2 messages, there are scenarios where a custom parser might be necessary. Custom parsers allow for greater flexibility and can handle unique or non-standard HL7v2 message formats.
Why Consider a Custom Parser?
- Unique Message Formats: Some healthcare systems might use HL7v2 message formats that deviate from the standard. A custom parser can be tailored to understand these unique formats.
- Specific Data Extraction: If there's a need to extract particular data elements from HL7v2 messages in a specific manner, a custom parser can be designed to do just that.
- Enhanced Error Handling: Custom parsers can be built with specialized error handling mechanisms to deal with known issues or quirks in the HL7v2 messages used by a specific healthcare system.
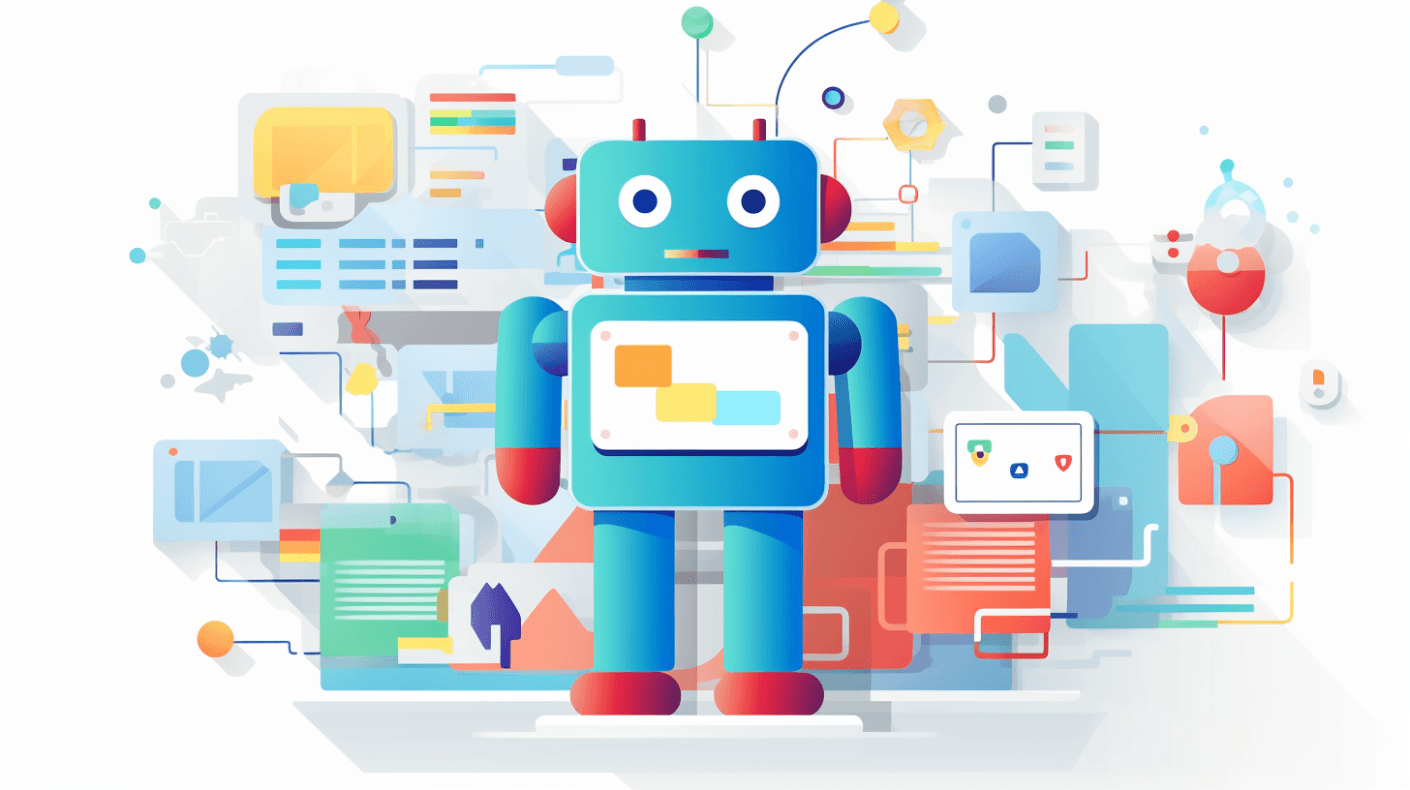
Building a Custom Parser
- Understand the Message Structure: Before building a custom parser, it's crucial to thoroughly understand the structure of the HL7v2 messages you'll be parsing. This involves identifying all segments, fields, and sub-components and understanding their significance.
- Define Parsing Rules: Determine how each segment, field, and sub-component should be parsed. This might involve specifying delimiters, recognizing data types, and handling repeating fields.
- Implement the Parser: Using a programming language of choice, implement the parser based on the defined rules. Popular languages for this task include Python, Java, and C#.
- Test the Parser: Before deploying the custom parser, test it extensively using real-world HL7v2 messages to ensure accuracy and reliability.
Integrating a Custom Parser with the Google Cloud Healthcare API
Once you've built a custom parser, you can integrate it with the Google Cloud Healthcare API to leverage its storage, management, and analysis capabilities.
- Host the Custom Parser: Deploy the custom parser on a server or cloud platform, ensuring it's accessible via an API endpoint.
- Send Messages to the Parser: Configure your healthcare system to send HL7v2 messages to the custom parser's endpoint for parsing.
- Store Parsed Messages: Once parsed, send the structured data to the Google Cloud Healthcare API for storage and further processing.
# Send HL7v2 message to custom parser for parsing
response = requests.post("https://custom-parser-endpoint.com/parse", data=message_content)
parsed_message = response.json()
# Store parsed message in Google Cloud Healthcare API
store_request = healthcare_v1beta1.StoreMessageRequest(name=hl7v2_store.name, message=parsed_message)
client.store_message(request=store_request)
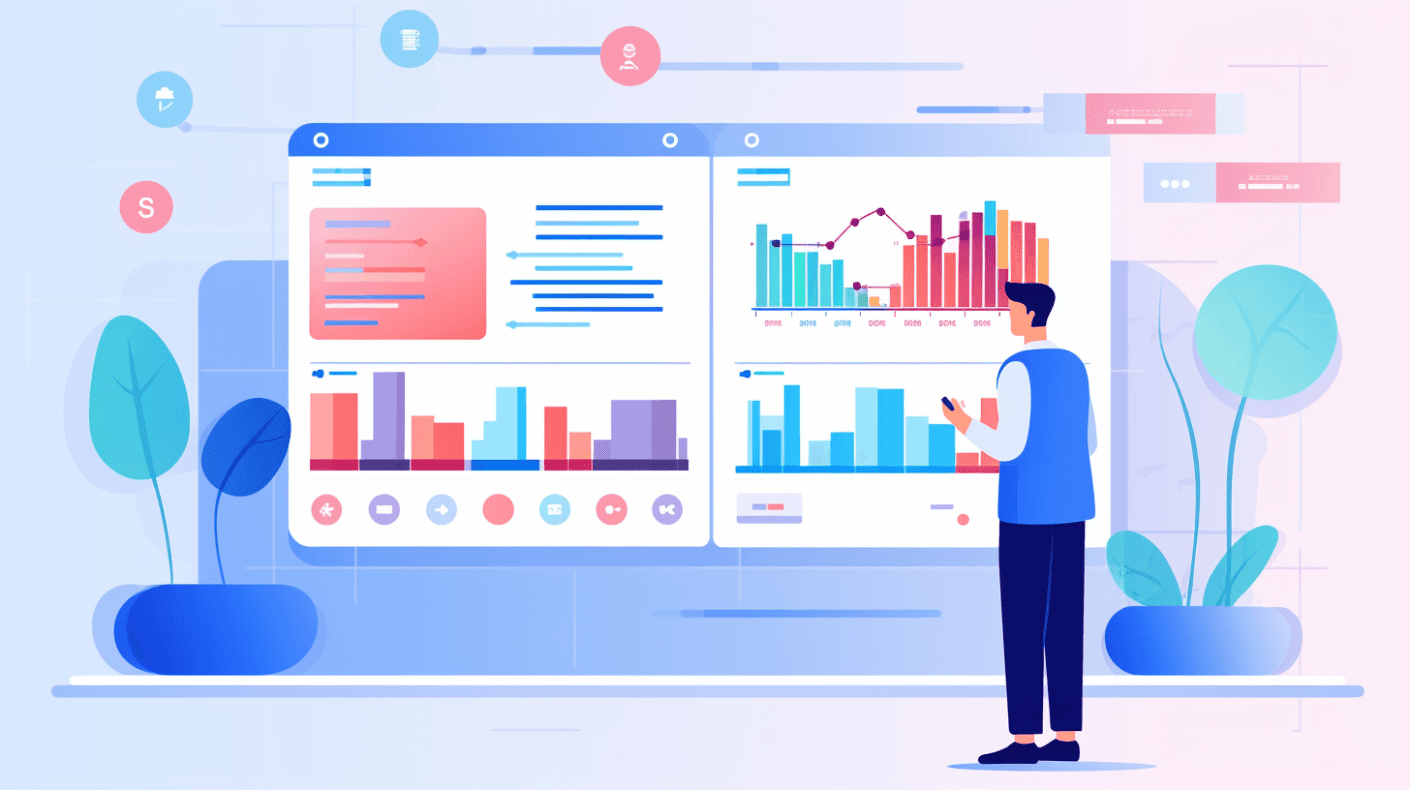
Maintaining a Custom Parser
Building a custom parser is just the beginning. It's essential to maintain and update the parser regularly to handle:
- Changes in Message Format: If the HL7v2 message format used by your healthcare system changes, the parser will need to be updated accordingly.
- Bug Fixes: If issues or inaccuracies are discovered in the parser, they should be addressed promptly.
- Performance Enhancements: As the volume of HL7v2 messages increases, the parser might need optimizations to handle the increased load.
Setting Up the MLLP Adapter for HL7v2 Messages
The MLLP (Minimal Lower Layer Protocol) adapter is an essential tool for healthcare systems that rely on HL7v2 messages. It facilitates the secure and reliable transmission of these messages over a network.
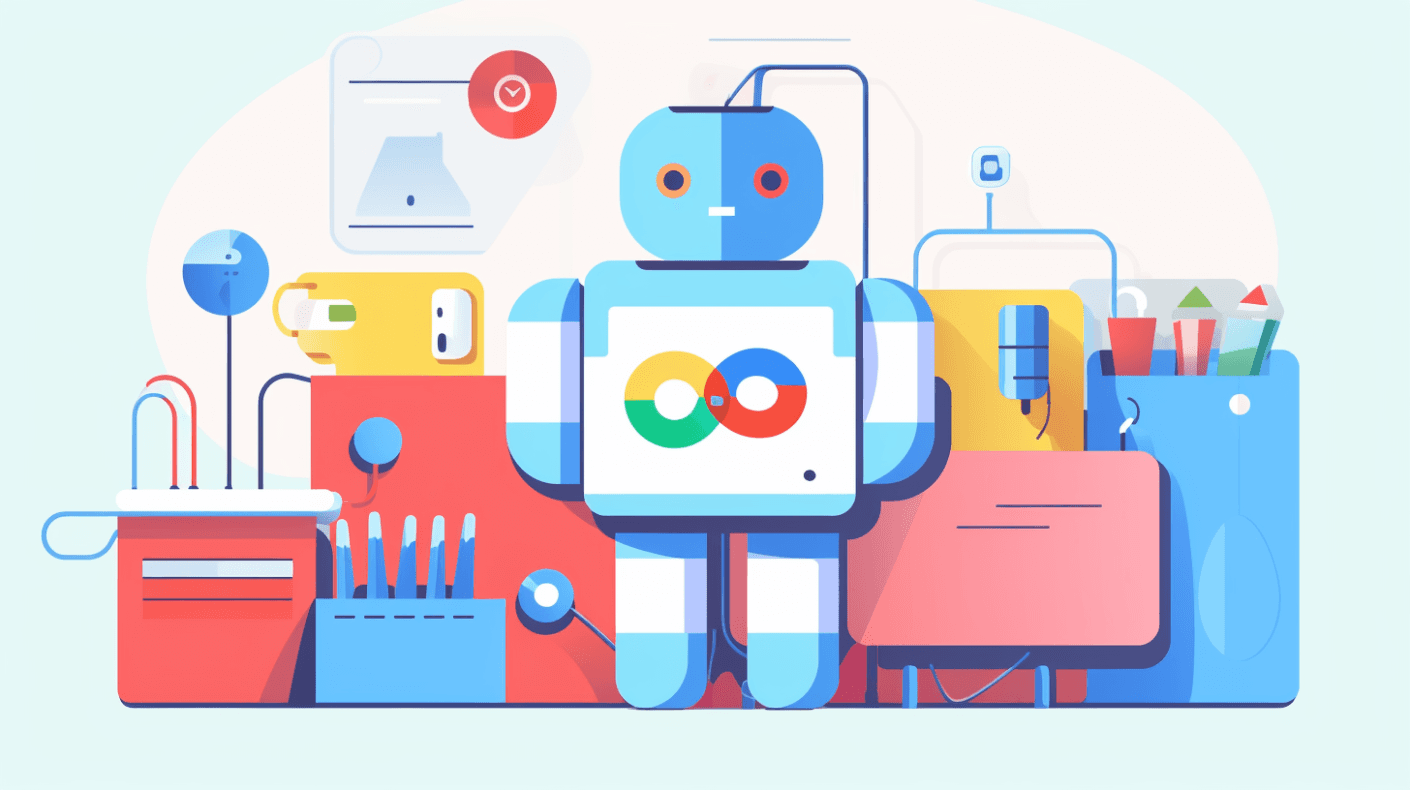
Understanding MLLP
- Purpose: MLLP is a data transport protocol used primarily in the healthcare industry for transmitting HL7 messages between systems.
- Reliability: MLLP ensures that messages are delivered reliably, with acknowledgments sent for received messages and mechanisms for handling transmission failures.
- Security: While MLLP itself doesn't provide encryption, it's often used in conjunction with secure protocols like TLS to ensure data privacy.
Setting Up the MLLP Adapter with Google Cloud Healthcare API
-
Installation
- The MLLP adapter is available as a Docker container, making it easy to deploy in various environments.
- Install Docker on your server or cloud platform.
- Pull the MLLP adapter container from the Google Container Registry.
docker pull gcr.io/cloud-healthcare-containers/mllp-adapter:latest
-
Configuration
- Before starting the MLLP adapter, you'll need to configure it to work with your specific environment and needs.
- This involves setting parameters like the port number, the HL7v2 store location, and any security settings.
-
Starting the Adapter
- Once configured, you can start the MLLP adapter using Docker.
docker run -p [PORT]:2575 gcr.io/cloud-healthcare-containers/mllp-adapter:latest --hl7v2_project_id=[PROJECT_ID] --hl7v2_location=[LOCATION] --hl7v2_dataset=[DATASET] --hl7v2_store=[STORE]
- Monitoring and Logging
- It's crucial to monitor the MLLP adapter to ensure it's operating correctly and efficiently.
- The adapter provides extensive logging capabilities, allowing you to track message transmissions, detect errors, and monitor performance.
Securing the MLLP Adapter
Given the sensitive nature of healthcare data, it's essential to secure the MLLP adapter to prevent unauthorized access and data breaches.
- TLS Encryption
- While MLLP doesn't encrypt data by default, the MLLP adapter supports TLS encryption.
- This ensures that HL7v2 messages are encrypted during transmission, protecting patient data.
- Authentication
- Implement authentication mechanisms to ensure that only authorized systems can send or receive messages via the MLLP adapter.
- This can involve using client certificates, IP whitelisting, or other authentication methods.
- Regular Updates
- Security threats evolve over time, so it's essential to regularly update the MLLP adapter to benefit from the latest security patches and enhancements.
Integration with Other Systems
The MLLP adapter can be integrated with various healthcare systems, EHRs (Electronic Health Records), and other tools. This ensures seamless data flow and enhances interoperability.
- Configuring Endpoints
- Ensure that the healthcare systems sending or receiving HL7v2 messages are correctly configured to communicate with the MLLP adapter.
- This might involve setting the correct IP address, port number, and any necessary authentication details.
- Message Transformation
- Depending on the systems you're integrating with, you might need to transform HL7v2 messages before transmission or after receipt.
- This can involve changing message formats, adding or removing fields, or converting messages to other standards like FHIR.
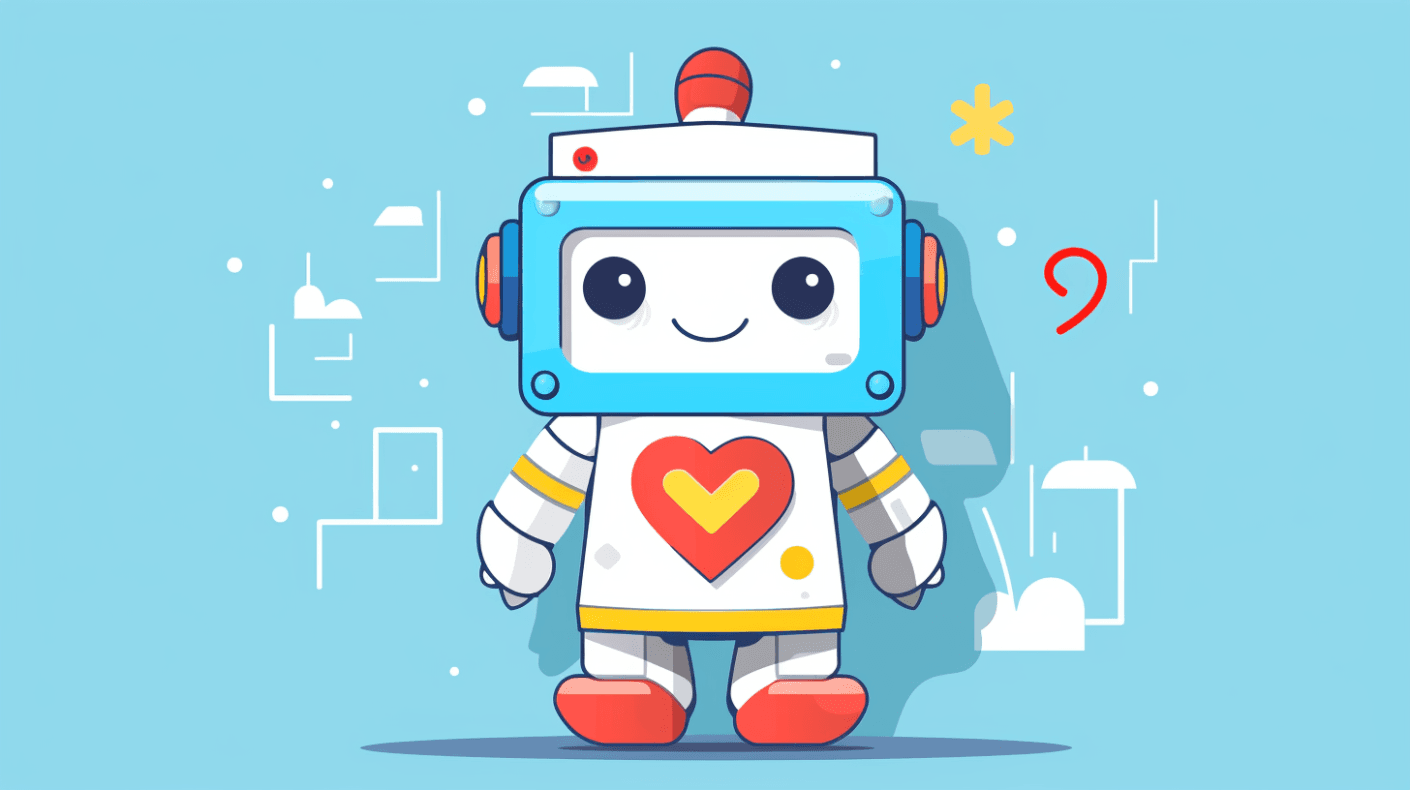
Sending HL7v2 Messages Over MLLP
Sending HL7v2 messages over MLLP is a standard practice in healthcare systems to ensure reliable and sequential data exchange. Here's a comprehensive guide to understanding and implementing this process:
Understanding the MLLP Transmission Process
- Start Block: Each message begins with a start block character, typically represented as
<SB>
. - Payload: This is the actual HL7v2 message content.
- End Block: Each message concludes with an end block character, usually represented as
<EB>
. - Acknowledgment: After receiving a message, the receiver sends back an acknowledgment. This can be either an acknowledgment of successful receipt (
ACK
) or a negative acknowledgment (NACK
) if there was an issue.
Steps to Send HL7v2 Messages Over MLLP
- Prepare the Message: Ensure the HL7v2 message is correctly formatted and includes all necessary segments and fields.
- Wrap the Message: Enclose the HL7v2 message with the MLLP start and end block characters.
- Establish a Connection: Connect to the receiving system using the designated IP address and port number.
- Transmit the Message: Send the wrapped HL7v2 message over the established connection.
- Receive Acknowledgment: Wait for the receiving system to send back an acknowledgment. Handle any negative acknowledgments by logging the error and potentially resending the message.
- Close the Connection: Once the transmission is complete and an acknowledgment is received, close the MLLP connection.
Tools & Libraries
There are several tools and libraries available that facilitate sending HL7v2 messages over MLLP:
- HAPI (HL7 Application Programming Interface): An open-source library that provides support for creating, parsing, and sending HL7 messages.
- Mirth Connect: A widely-used integration engine that supports HL7 message transmission over MLLP.
Forwarding HL7v2 Messages to a URL Over HTTPS
Once HL7v2 messages are received, they often need to be forwarded to another system or service for further processing. HTTPS provides a secure method for this forwarding, ensuring data privacy and integrity.
Benefits of Using HTTPS
- Security: HTTPS encrypts the data during transmission, ensuring that sensitive patient data remains confidential.
- Data Integrity: HTTPS ensures that the data is not altered during transmission.
- Authentication: The receiving system can verify the identity of the sender, ensuring data is only received from trusted sources.
Steps to Forward HL7v2 Messages Over HTTPS
- Prepare the Endpoint: Ensure the receiving system has an HTTPS endpoint set up to receive the HL7v2 messages.
- Format the Message: Convert the HL7v2 message into a format suitable for HTTPS transmission, such as JSON or XML.
- Establish a Secure Connection: Using an HTTPS client, establish a secure connection to the receiving system's endpoint.
- Send the Message: Transmit the formatted HL7v2 message over the established HTTPS connection.
- Handle the Response: The receiving system will typically send back a response. This could be a simple acknowledgment or more detailed feedback. Handle this response appropriately, logging any errors or issues.
- Close the Connection: After transmission and response handling, close the HTTPS connection.
Tools & Libraries
Several tools and libraries can assist in forwarding HL7v2 messages over HTTPS:
- Postman: A popular tool for API testing and development. It can be used to send HL7v2 messages to an HTTPS endpoint.
- cURL: A command-line tool for sending data over various protocols, including HTTPS.
- Libraries: Many programming languages have libraries or modules that support HTTPS communication, such as Python's
requests
library or Java'sHttpURLConnection
.