Mirth Connect: Part 1 - Getting Started with 🐳 Docker
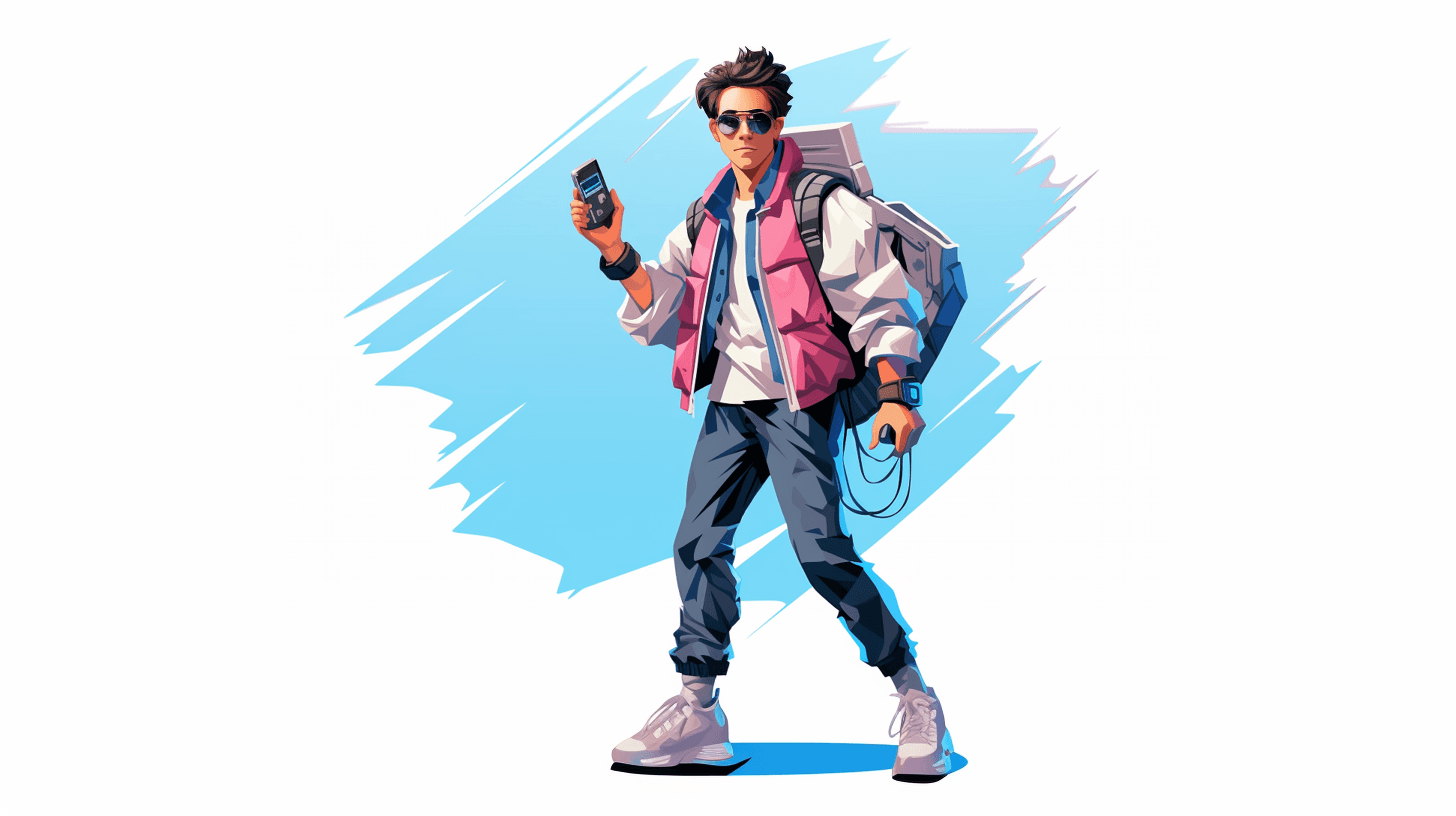
Getting Started
The first thing you’ll need to do is head over to Docker and download the application for your particular OS.
Once Docker is installed and running, pull down the latest NextGen Connect Docker image with: docker pull nextgenhealthcare/connect
You should see an image with repository: nextgenhealthcare/connect
and tag 'latest'.
Finally, you can start a Connect container by simply running: docker run --name connect -d -p 8443:8443 nextgenhealthcare/connect
-name connect
names your containerconnect
d
runs your container in the backgroundp 8443:8443
maps your OS port 8443 to the container's port 8443nextgenhealthcare/connect
tells docker what image to use, in this case the latest image, when creating your container
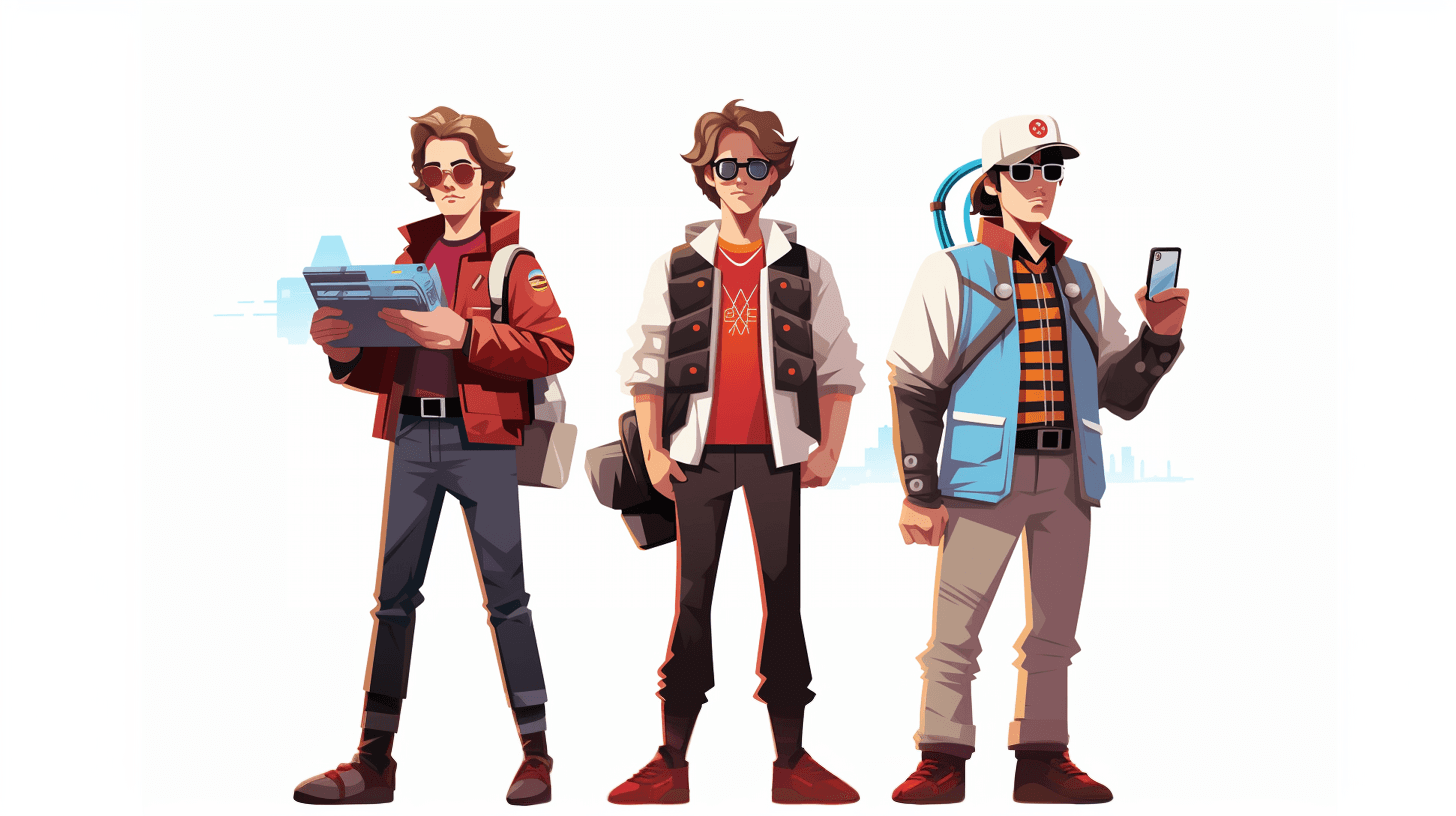
At this point Connect is running and you can interact with it as you normally would (example: open a browser with [https://localhost:8443](https://localhost:8443/))
.
It’s important to note that only port 8443 has been mapped to the host OS so sending a message to a channel you’ve deployed on Connect running in this container will fail until you map ports for your channels.
You can check the status of this container with: docker ps -a
And you can check the server logs with: docker logs connect
When you are done, you can stop and remove the container with: docker stop connect && docker rm connect
Please note the following when starting, stopping, and removing a simple Connect container running on the embedded Derby database: When the Connect container is removed, so is your database, which includes all of your channels and messages. Head to the "Docker Volumes" section to learn about persisting the data.
Configuring
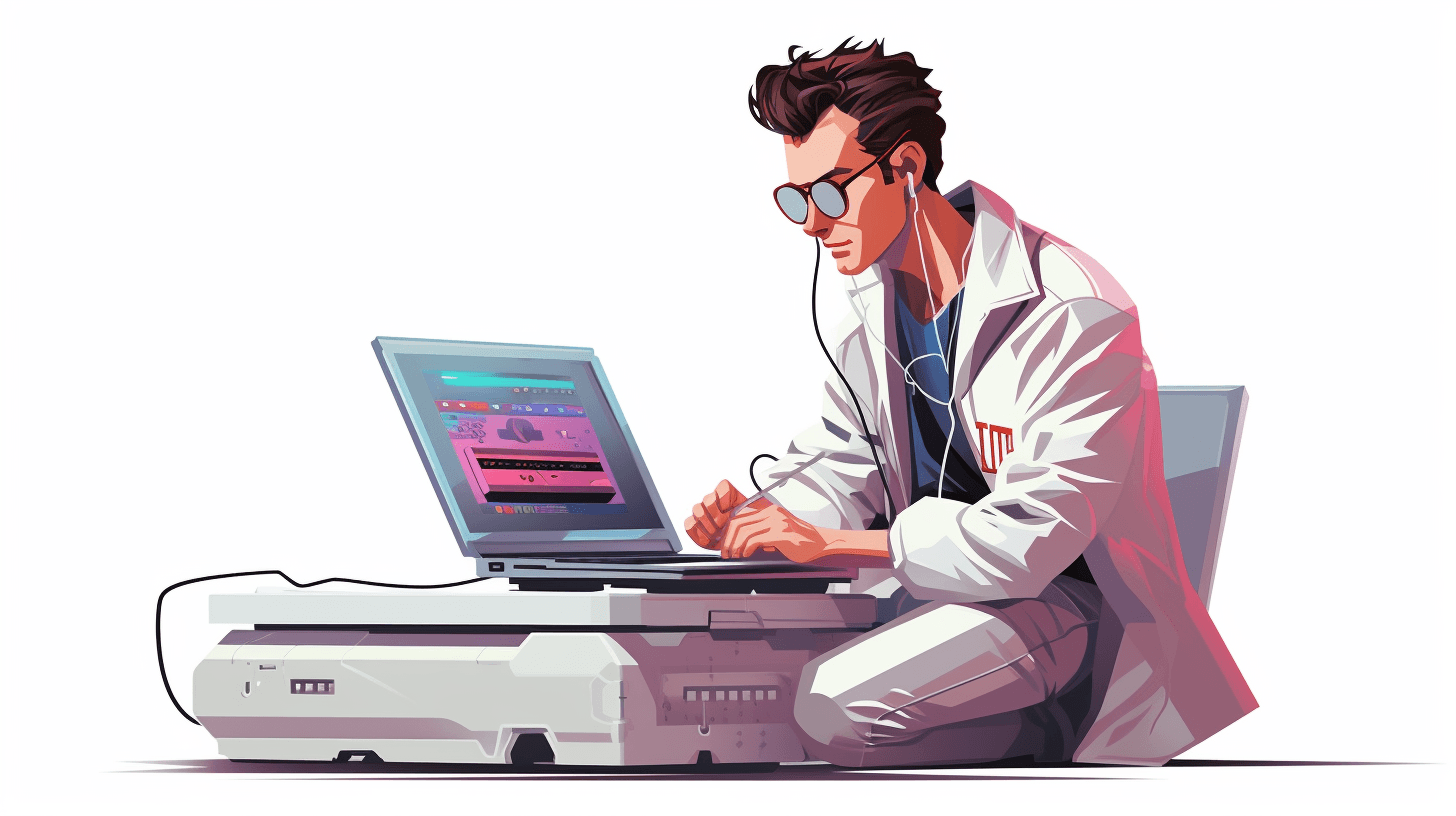
So now you are asking yourself, well that’s all well and good, but how do I use a different database? And how do I set my keystore password? Or even change the VM options? We’ve designed Connect Docker so that it can be configured in several ways. The first is by use of environment variables.
Environment Variables
Our Docker images natively support the following environment variables:
DATABASE (ex: derby, postgres, mysql, oracle, sqlserver)
DATABASE_URL
DATABASE_USERNAME
DATABASE_MAX_CONNECTIONS
KEYSTORE_STOREPASS
KEYSTORE_KEYPASS
SESSION_STORE
VMOPTIONS
DELAY
To start a Connect container using one or more environment variables you use the -e parameter. For instance, to specify what type of database to use and set a VM option you could do the following: docker run --name connect -e DATABASE='derby' -e VMOPTIONS='-Xmx512m' -p 8443:8443 nextgenhealthcare/connect
Most of the environment variables offer an easy way to get custom properties into Connect server’s mirth.properties file. As you may know, there are a ton of optional properties that can go into this file (For a full list, search the User Guide for “mirth.properties”), so how do you configure all of the others?
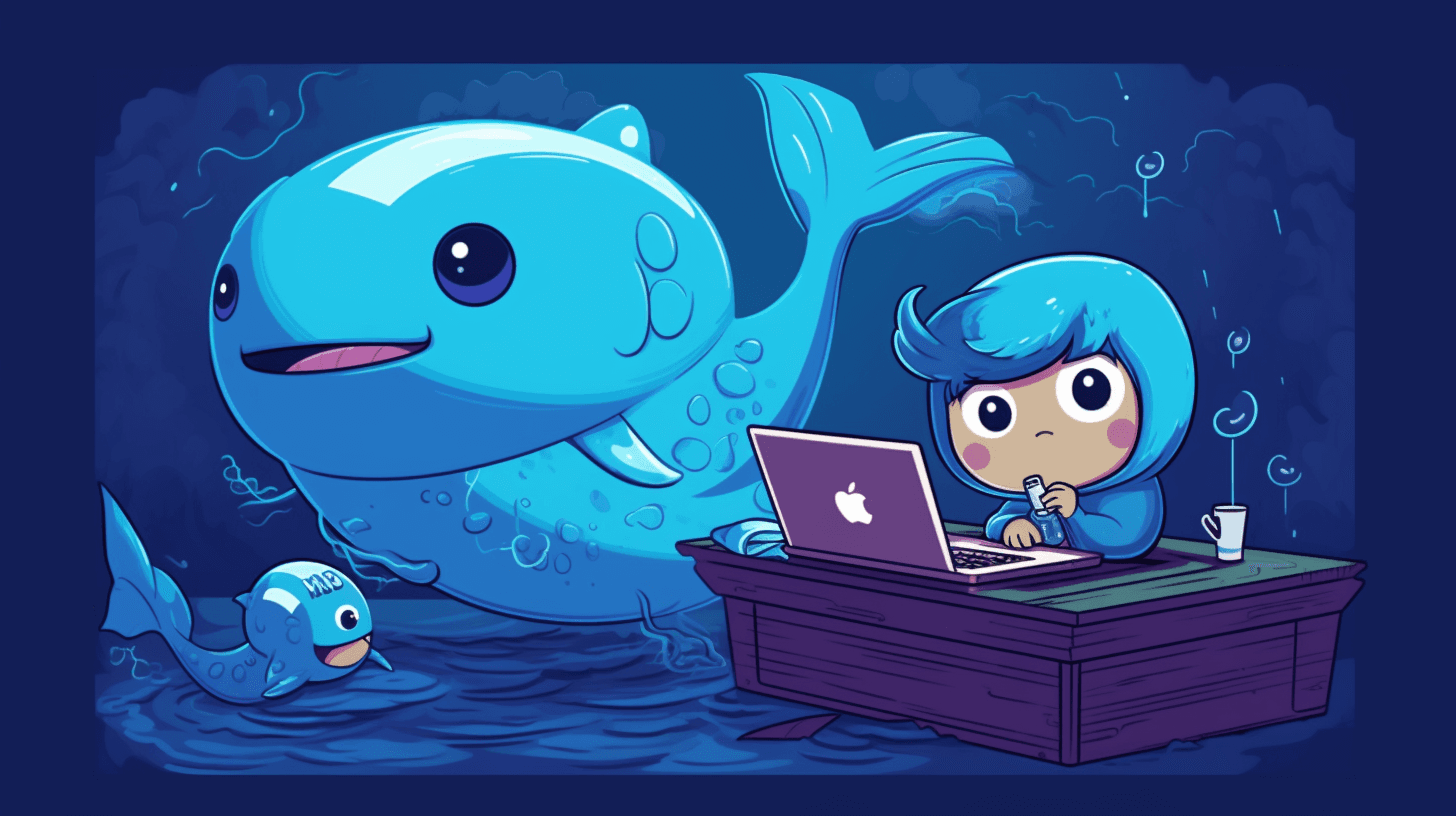
We added a system that allows you to add any arbitrary property. To do this you would use a special environment variable syntax where you start with _MP_
then replace -
with _
then replace .
with __
. Here’s an example of how to add the property database-readonly.max-connections = 25
: docker run --name connect -e _MP_DATABASE__READONLY_MAX__CONNECTIONS='25' -p 8443:8443 nextgenhealthcare/connect
Adding all of your environment variables as command line arguments can get pretty tedious and verbose but Docker supports loading environment variables from a file.
Here’s an example file (be sure to substitute serverip
with the correct IP): myenvfile.txt
DATABASE=postgres
DATABASE_URL=jdbc:postgresql://serverip:5432/mirthdb
DATABASE_USERNAME=postgres
DATABASE_PASSWORD=postgres
KEYSTORE_STOREPASS=changeme
KEYSTORE_KEYPASS=changeme
VMOPTIONS=-Xmx512m
And Docker using the file: docker run --env-file=myenvfile.txt -p 8443:8443 nextgenhealthcare/connect
Docker Secrets
You may have noticed that we are passing usernames and passwords as Docker parameters in the command line. There is a more secure way of doing this using Docker Secrets. Docker Secrets are encrypted during transit and at rest in a Docker Swarm and Secrets are only accessible to services that have been granted explicit access and only while running. Docker secrets can be used in Docker Swarm, Docker Compose, and Docker Stack.
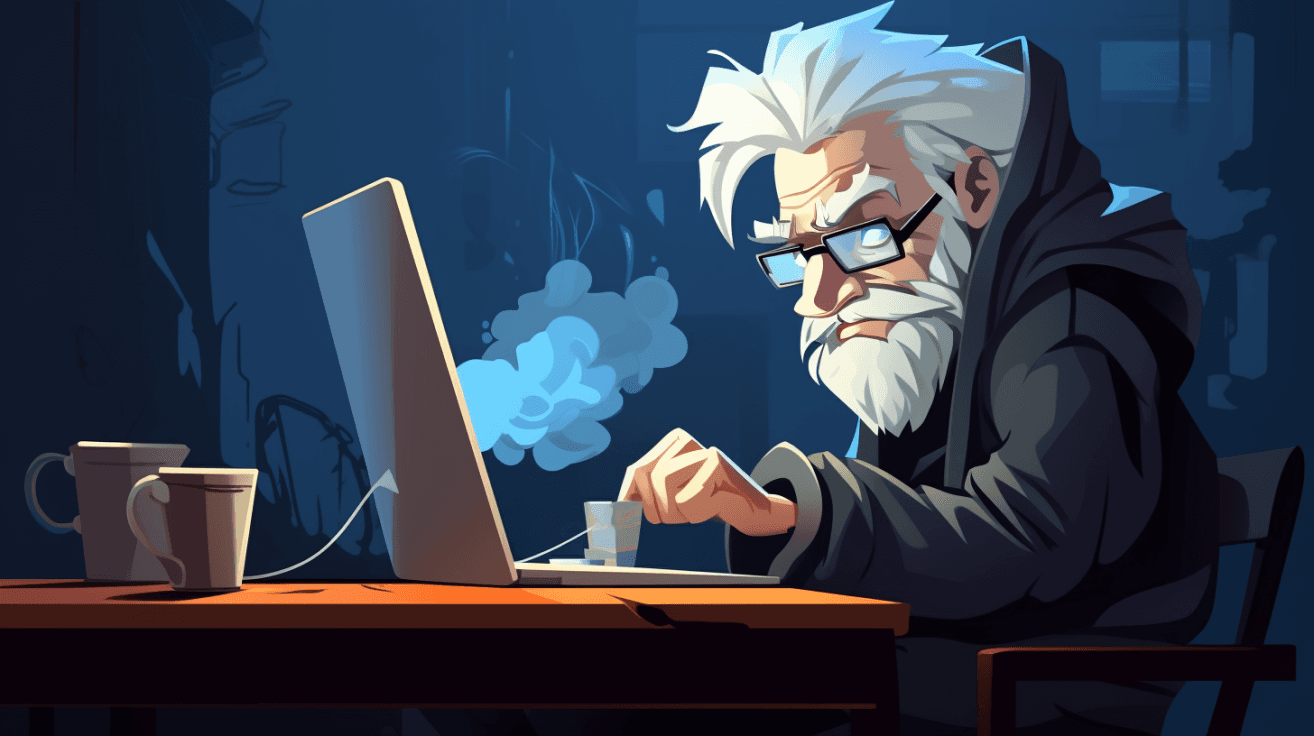
The Connect Docker images support the two secret names: mirth_properties
and mcserver_vmoptions
. Properties in the mirth_properties
secret will be merged into the container’s mirth.properties
file and VM options in the mcserver_vmoptions
secret will be added to the container’s mcserver.vmoptions
file. The following example shows how to use Docker Compose to bring up Connect with a mirth_properties
secret.
First create a secret file: secret.properties
keystore.storepass=changeme
keystore.keypass=changeme
Now define your stack in a YAML file (be sure to edit the path to the secret.properties file on your file system): mirth_stack.yml
version: '3.1'
services:
mc:
image: nextgenhealthcare/connect
container_name: connect
secrets:
- mirth_properties
ports:
- 8080:8080/tcp
- 8443:8443/tcp
secrets:
mirth_properties:
file: /local/path/to/secret.properties
With your two files created you can now use Docker Compose to deploy: docker-compose -f mirth_stack.yml -d up
Once Connect has started in the container you can see that the secret properties have been merged by running: docker exec -it connect cat conf/mirth.properties
To bring down the stack: docker-compose -f mirth_stack.yml down
As you continue down the path you may want to leverage git and some automated testing, thankfully a couple common tools have been made available.
Version Control
Mirth Sync: https://github.com/SagaHealthcareIT/mirthsync
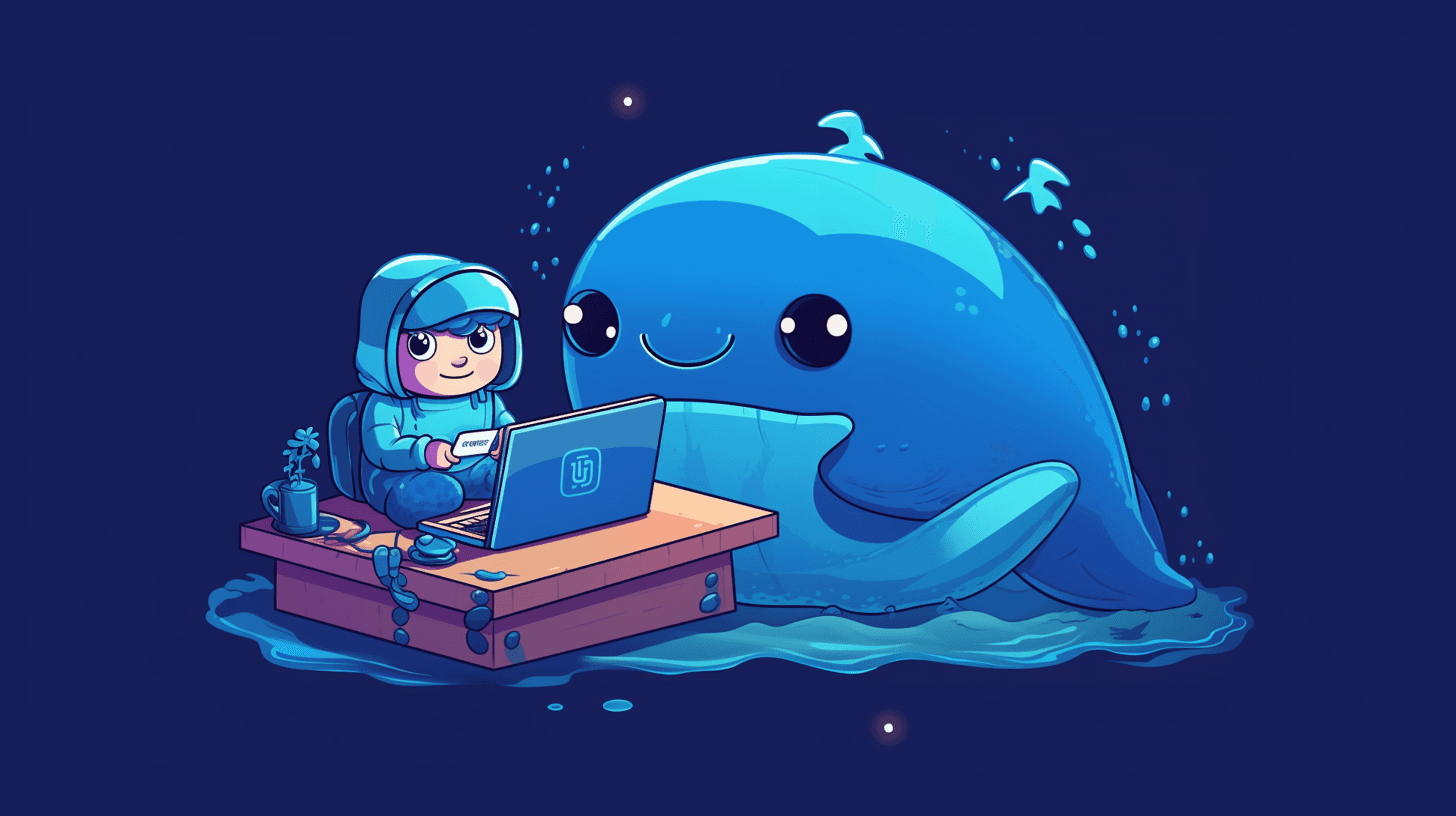
Mirth Sync is a command line tool for synchronizing Mirth Connect code between servers by allowing you to push or pull channels, code templates, configuration map and global scripts. The tool can also be integrated with Git or other version control systems for the purpose of tracking changes to Mirth Connect code and configuration.
Git-ext: https://github.com/kayyagari/git-ext
Git Ext is a MirthConnect extension for version controlling Channels and CodeTemplates. Git is used as the VCS on the server-side using JGit.
Automated Testing
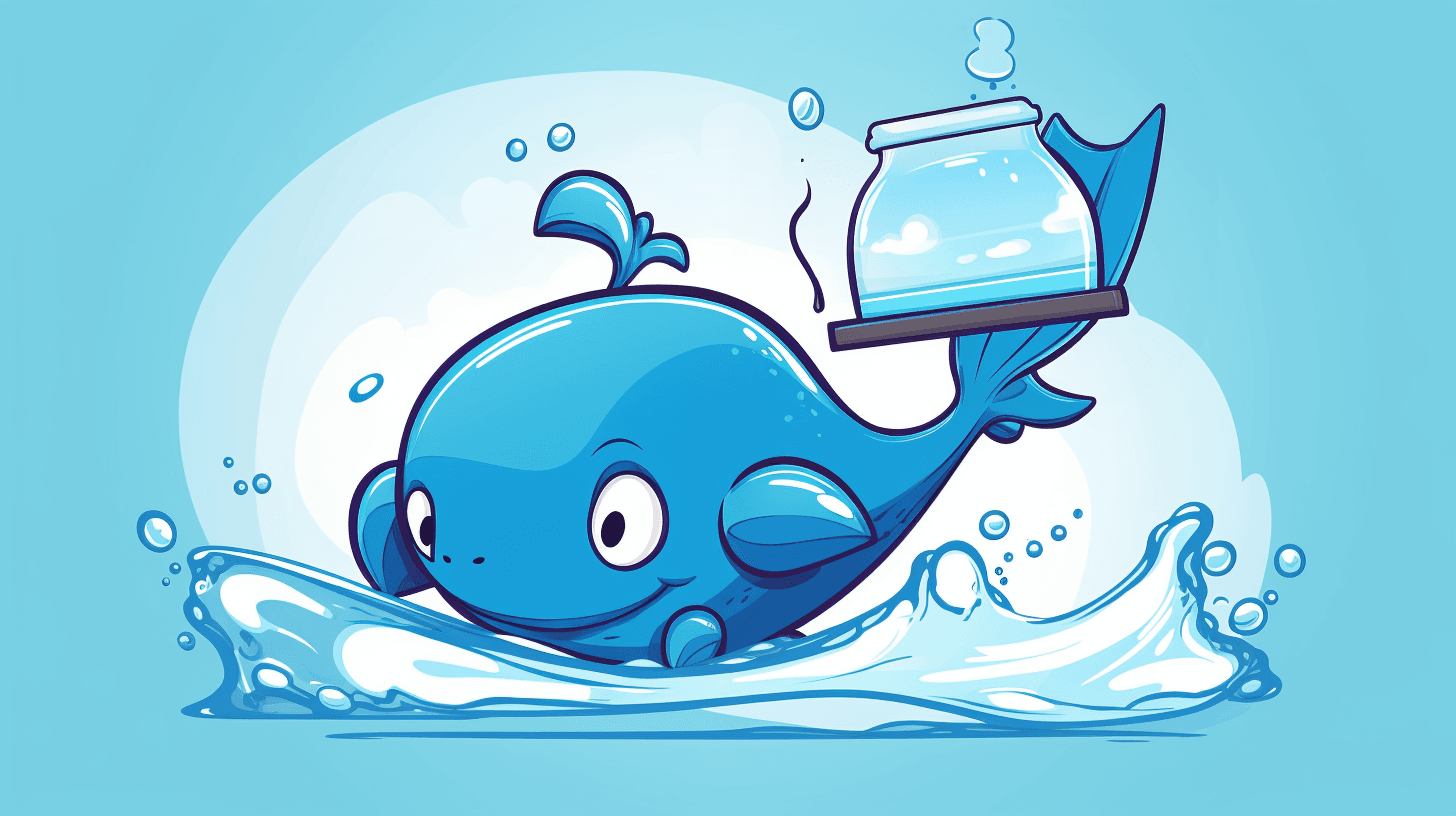
Jest unit testing framework for mirth: https://github.com/vibinernesto/Mirth-Jest-Framework
Docker Hygiene Cheat Sheet
As you work with Docker, however, it’s also easy to accumulate an excessive number of unused images, containers, and data volumes that clutter the output and consume disk space.
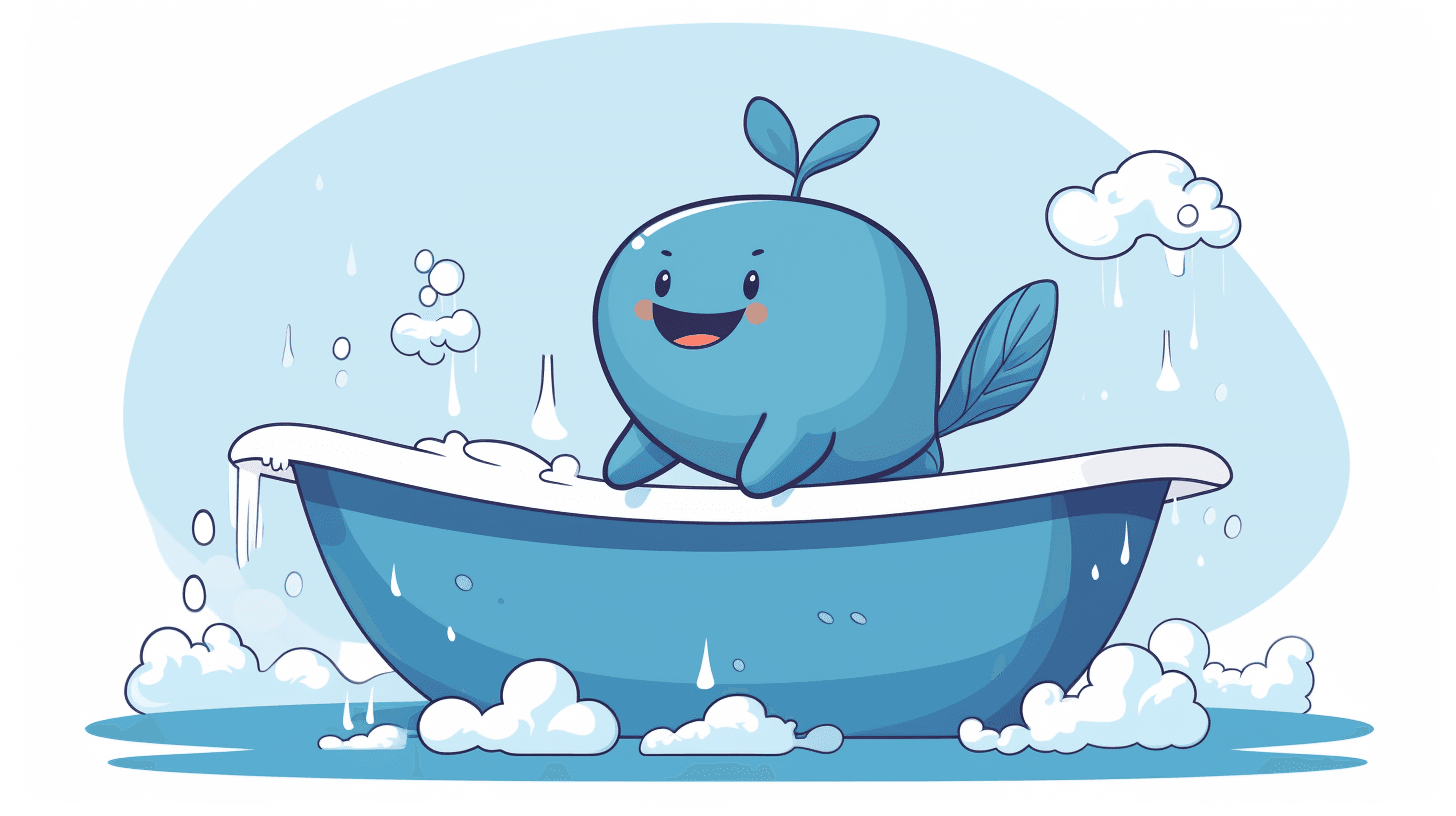
# Docker provides a single command that will clean up any resources — images,
# containers, volumes, and networks — that are dangling (not tagged or associated
# with a container).
docker system prune
# To additionally remove any stopped containers and all unused images (not just
# dangling images), add the -a flag to the command.
docker system prune -a
# Use the docker images command with the -a flag to locate the ID of the images
# you want to remove. This will show you every image, including intermediate
# image layers. When you’ve located the images you want to delete, you can pass
# their ID or tag to docker rmi.
docker images -a
docker rmi Image Image
# Remove Dangling Images
docker images -f dangling=true
docker image prune
# Remove By Pattern
docker images -a | grep "pattern"
docker images -a | grep "pattern" | awk '{print $3}' | xargs docker rmi
# Remove all images
docker images -a
docker rmi $(docker images -a -q)
# Remove Containers
docker ps -a
docker rm ID_or_Name ID_or_Name
# Remove all exited containers
docker ps -a -f status=exited
docker rm $(docker ps -a -f status=exited -q)
# Remove all containers with multiple filters
docker ps -a -f status=exited -f status=created
docker rm $(docker ps -a -f status=exited -f status=created -q)
# Stop and remove all containers
docker ps -a
docker stop $(docker ps -a -q)
docker rm $(docker ps -a -q)
# Remove volumes
docker volume ls
docker volume rm volume_name volume_name
# Remove dangling volumes
docker volume ls -f dangling=true
docker volume prune
# Remove a container and its volume
docker rm -v container_name
# Remove all containers according to a pattern
# You can find all the containers that match a pattern using a
# combination of `docker ps` and `grep`.
# When you’re satisfied that you have the list you want to delete,
# you can use `awk` and `xargs` to supply the ID to `docker rm`.
# Note that these utilities are not supplied by Docker and are
# not necessarily available on all systems:
docker ps -a | grep "pattern”
docker ps -a | grep "pattern" | awk '{print $1}' | xargs docker rm