🫧 React State Management & Clean SPA Architecture
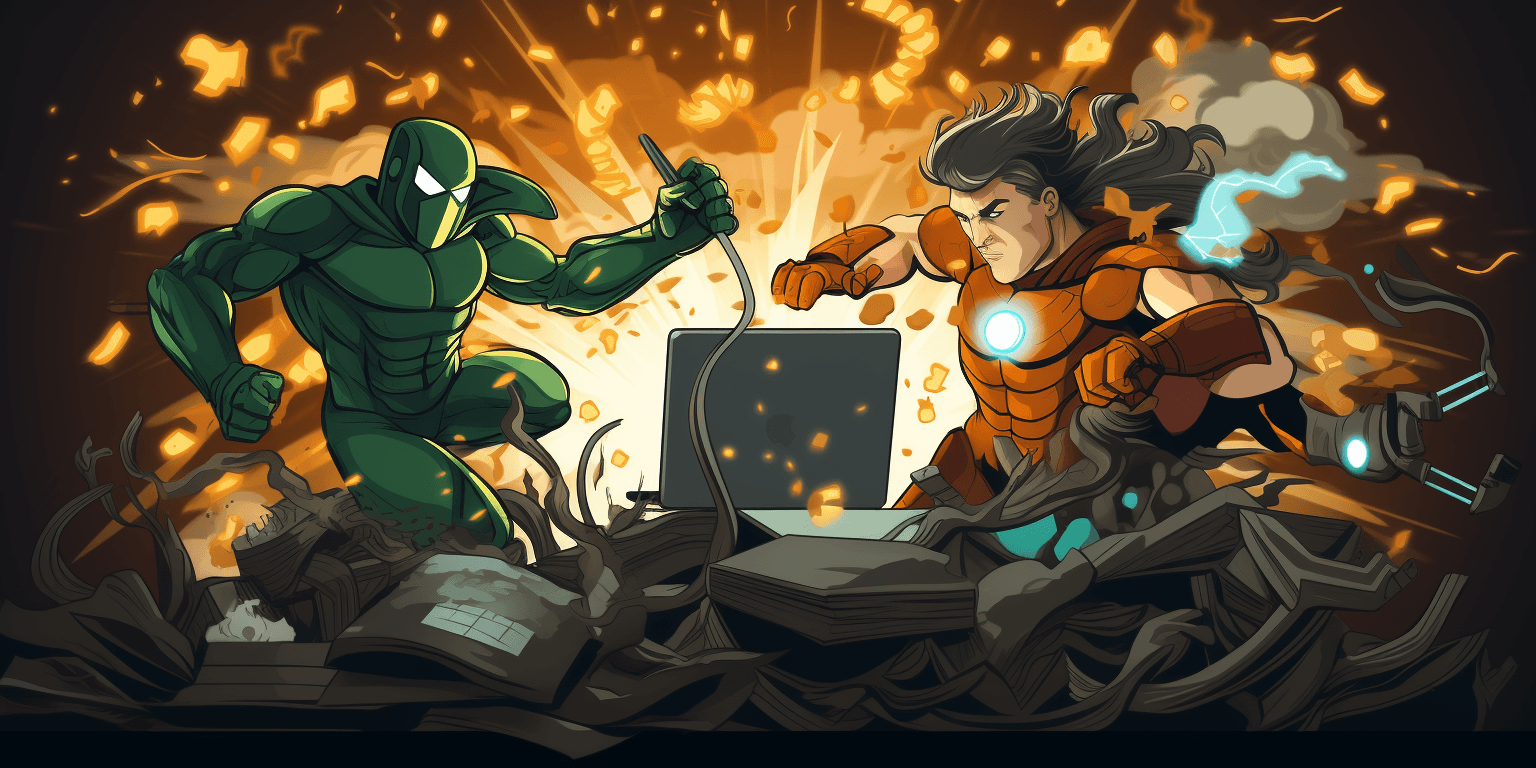
When state management matters
TL;DR - State management becomes much less important when working with SSR, but it's still important to understand the basics of state management in React.js. Chances are you may see a React.js single page application with a need for simple state management. In this article, we will explore the most popular state management tools in the React.js ecosystem: Redux, Zustand, Recoil, and the Context API, but there are many more.
Prop Drilling
When working with a single page application, prop drilling between components can become a headache, here are some ways to simplify your state management. Prop drilling is a basic approach where data is passed down through the component tree as props. It's a simple and native way to manage state, but it has limitations:
- Limited to Parent-Child Relationships: Prop drilling works well for passing data between parent and child components but can become cumbersome in deeply nested components.
- Complexity: As your component tree grows, you might find yourself passing props through many intermediate components, leading to increased complexity.
- Prop drilling is suitable for smaller applications or for passing data between closely related components.
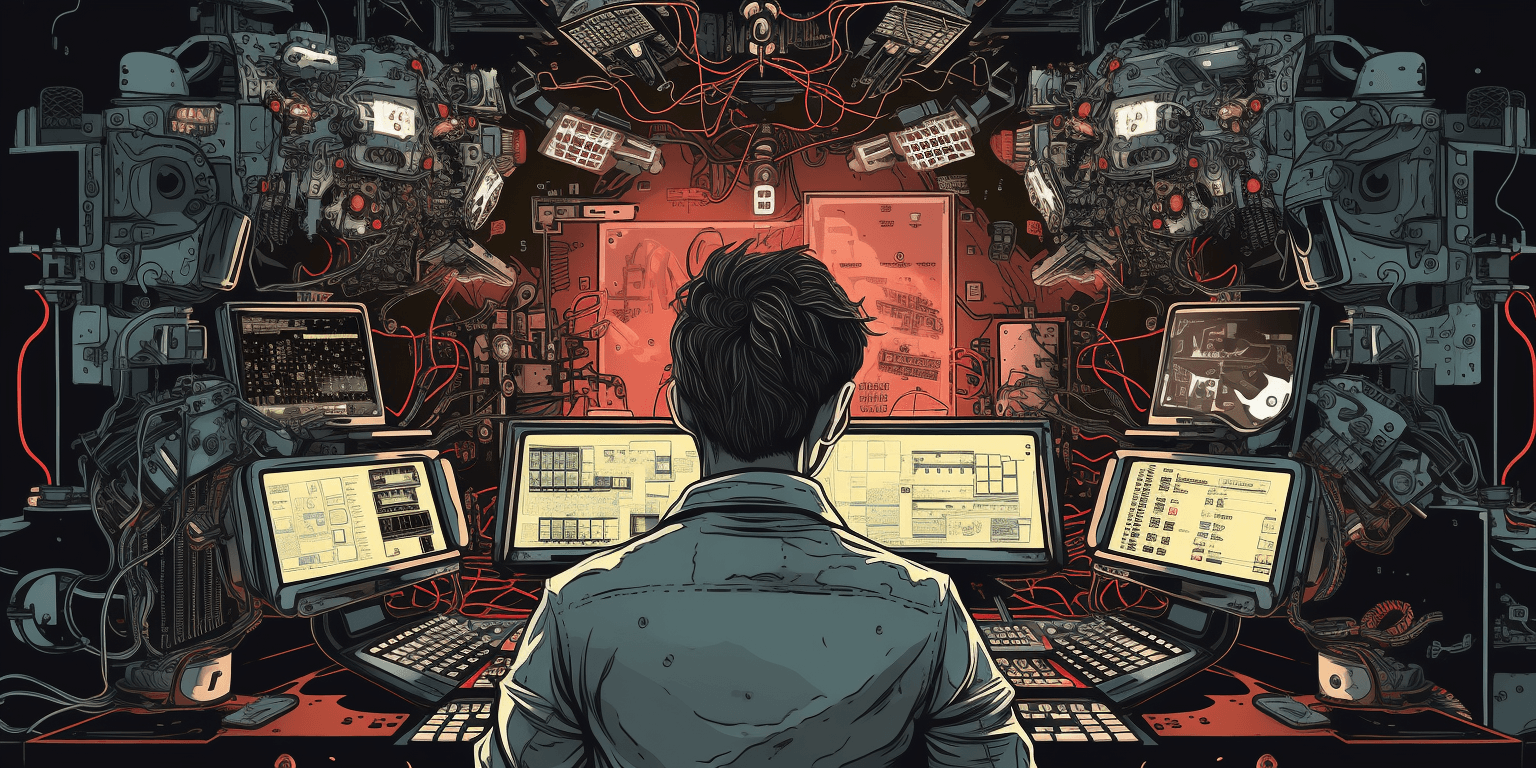
Understanding State Management in React.js
Before diving into the specifics of each tool, let's establish a solid understanding of state management in React.js. State refers to the data that controls the behavior and appearance of a component. React components have both local state and global state. Local state is confined to a single component, while global state is shared across multiple components.
State management is a crucial aspect of building robust and scalable React applications. It allows developers to handle dynamic data and ensure that components remain in sync with each other. By effectively managing state, we can create more efficient and maintainable code.
Local state is used when a component needs to manage its own data. For example, a form component may have a local state to keep track of user input. On the other hand, global state is used when multiple components need to share and synchronize data. This is often achieved using libraries like Redux or React Context API.
The Importance of State Management
Effective state management plays a fundamental role in React.js development. It allows us to keep track of data that changes over time and ensures that all components stay synchronized. By centralizing state management, we can simplify our codebase and reduce bugs caused by inconsistent or redundant data.
When state is managed properly, it becomes easier to reason about the application's behavior. Developers can easily trace the flow of data and understand how different components interact with each other. This leads to better code maintainability and makes it easier to introduce new features or fix bugs.
Furthermore, state management is crucial for building scalable applications. As the complexity of an application grows, managing state becomes more challenging. With proper state management techniques, we can handle large amounts of data and ensure that our application remains performant.
Core Concepts of State Management
When it comes to state management, some core concepts are worth highlighting. First and foremost, immutability is crucial. By keeping state immutable, we avoid unintended side effects and make it easier to track changes. Immutable data ensures that state updates are predictable and helps prevent bugs caused by accidentally modifying the state.
Actions, reducers, and selectors are key elements in state management. Actions represent events that cause state changes. They are dispatched by components or other parts of the application to trigger updates. Reducers specify how state changes in response to actions. They are responsible for updating the state based on the dispatched actions. Selectors allow components to access specific parts of the state. They provide a way to extract data from the state without directly accessing it.
By following these core concepts, we can build a robust and scalable state management system in React.js. Understanding how actions, reducers, and selectors work together is essential for effectively managing state and creating maintainable code.
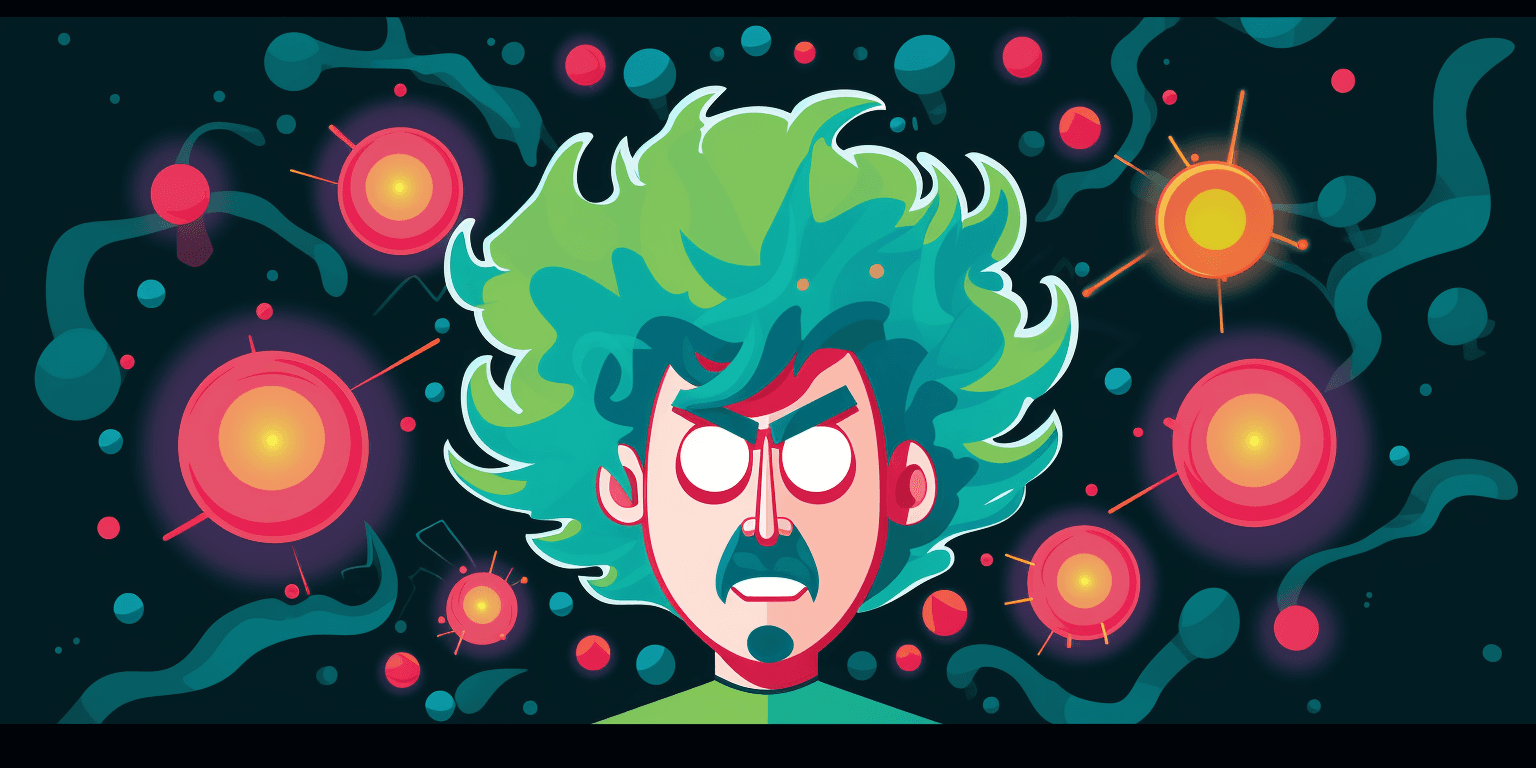
An Overview of Redux
Redux is one of the most established state management libraries in the React.js ecosystem. It follows a centralized approach, making it suitable for large-scale applications with complex state requirements.
Redux provides a predictable state container, enabling efficient debugging by maintaining a log of all state changes. This log can be accessed to track the flow of data and identify any issues that may arise during development.
One of the key features of Redux is its ability to offer a single source of truth. This means that the entire application's state is stored in a single JavaScript object. By having a centralized state, Redux ensures that every component has access to the same state, eliminating the need for passing props between deeply nested components. This simplifies the development process and makes it easier to manage and update the state of the application.
Another advantage of using Redux is the vast ecosystem of middleware and plugins available. These extensions provide additional functionality and enhance the capabilities of Redux. Developers can choose from a wide range of options to customize and optimize their state management solution.
Key Features of Redux
Redux offers a variety of features to facilitate state management. One of its key features is a single source of truth, where the entire application's state is stored in a single JavaScript object. This ensures that every component has access to the same state and eliminates the need for passing props between deeply nested components. Redux also provides a predictable state container, enabling efficient debugging by maintaining a log of all state changes.
Additionally, Redux supports time-travel debugging, which allows developers to replay past actions and observe how the state of the application evolves over time. This feature is particularly useful when debugging complex applications with intricate state interactions.
Another notable feature of Redux is its support for middleware. Middleware functions can be added to the Redux store to intercept and modify actions before they reach the reducers. This allows developers to implement additional logic, such as asynchronous operations or logging, in a clean and organized manner.
Pros and Cons of Using Redux
While Redux offers many advantages, it may not be suitable for all projects. One of its notable benefits is the vast ecosystem of middleware and plugins available, which extends its functionality. Developers can choose from a wide range of options to enhance their state management solution and tailor it to their specific needs.
However, one of the challenges of using Redux is its steeper learning curve compared to other state management tools. The concepts and patterns used in Redux, such as actions, reducers, and the store, may be unfamiliar to developers who are new to the library. This initial learning curve can be daunting, but once mastered, Redux can greatly simplify state management and improve the overall structure of the application.
Another potential drawback of Redux is its verbosity. Redux requires more code compared to other alternatives, which can lead to increased development time and effort. However, the benefits of having a centralized state and a predictable state container often outweigh the additional code required.
In conclusion, Redux is a powerful state management library that offers a centralized approach and a predictable state container. It provides a single source of truth for the application's state, simplifying the development process and making it easier to manage and update the state. While Redux may have a steeper learning curve and require more code compared to other alternatives, its vast ecosystem of middleware and plugins, along with its debugging capabilities, make it a popular choice for managing state in large-scale React.js applications.
Diving into Zustand
Zustand is relatively new but quickly gaining popularity among React.js developers. It is praised for its simplicity and developer-friendly syntax.
Understanding Zustand's Functionality
Zustand operates based on a hook-oriented paradigm, making it easy to integrate into existing React.js codebases. It leverages React's context API and provides hooks for accessing and updating the shared state. Zustand encourages a more lightweight and flexible approach to state management without sacrificing performance.
Strengths and Weaknesses of Zustand
One of Zustand's strengths is its small bundle size, making it an excellent choice for performance-critical applications. Additionally, Zustand offers a straightforward API, reducing the learning curve for developers. However, it lacks some advanced features available in other state management libraries, which may limit its applicability in complex use cases.
Exploring Recoil
Recoil, introduced by Facebook, aims to provide an intuitive and efficient way to manage global state in React.js applications.
What Makes Recoil Unique?
Recoil distinguishes itself by its focus on the concept of atoms, which represent individual units of state. These atoms can then be combined to create derived and shared state across components. Recoil embraces a declarative approach, allowing developers to write state management logic using React components themselves.
Advantages and Disadvantages of Recoil
Recoil offers several advantages, such as an easy learning curve for developers familiar with React.js and a highly flexible and scalable architecture. It also provides a powerful snapshot feature that enables time-travel debugging and caching optimization. On the other hand, Recoil is still relatively new compared to other established state management libraries, which means it may have a smaller ecosystem and fewer community resources.
The Context API in React.js
The Context API is a built-in feature in React.js that provides a simple way to share state between components without using third-party libraries.
The Basics of Context API
The Context API operates on a provider-consumer model, where a provider component wraps a subtree of components that need access to the shared state. By consuming this context, components can gain direct access to the state provided by the nearest provider in the component hierarchy.
Evaluating the Context API: Benefits and Drawbacks
The Context API offers a lightweight solution for managing state within small to medium-sized applications. It requires no additional dependencies and has a shallow learning curve, making it an appealing choice for simple use cases. However, as the application grows and the state becomes more complex, the Context API may not scale as efficiently as other dedicated state management tools.
As React.js continues to evolve, so too do the options for state management. Redux, Zustand, Recoil, and the Context API each have their strengths and weaknesses, and choosing the right one depends on the specific needs of your project. Whether you prefer a centralized approach with Redux or a more lightweight solution with Zustand or Recoil, always consider the trade-offs and evaluate which tool best aligns with your development goals. By leveraging the power of these state management tools, you can ensure a well-structured and efficient React.js application.